WPF 制作电子相册浏览器
标签:比较 linq int cli 手动 lin ret blog osi
原文:WPF 制作电子相册浏览器
周末的时候,闲着无聊,做了一个电子相册浏览器。比较简单。界面如下:
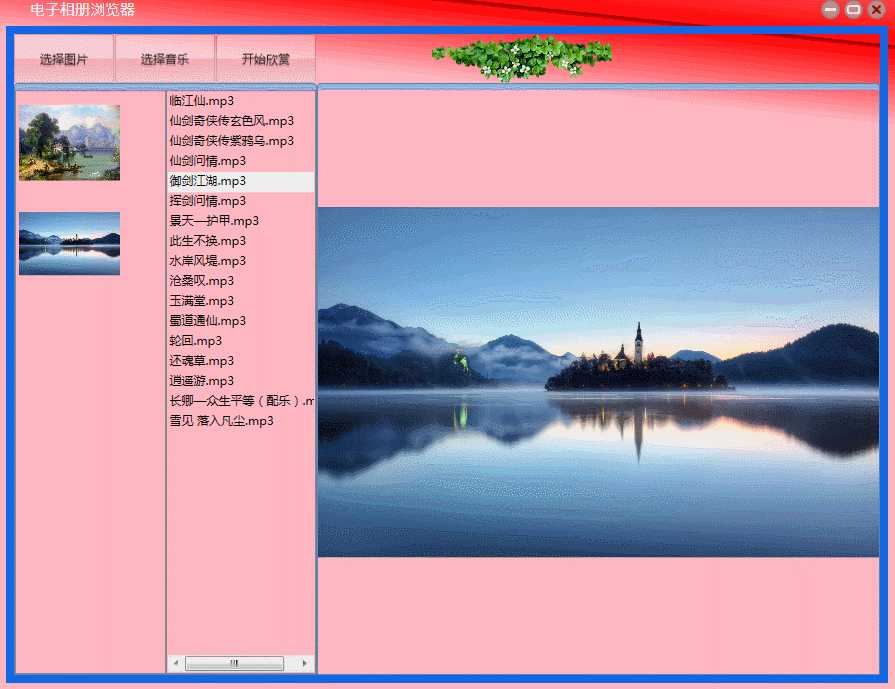
主要部分代码如下:
MainWindow.xaml
MainWindow.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Windows.Forms;
using System.Windows.Threading;
using System.Drawing;
using System.Xml.Linq;
namespace PictureMagic
{
///
/// MainWindow.xaml 的交互逻辑
///
public partial class MainWindow : HeaderedWindow
{
public MainWindow()
{
InitializeComponent();
m_picureInfoList = new List();
m_musicList = new List();
this.picShow.DataContext = m_picutureInfoViewModel;
Timer_Init();
InitShowImageTimer();
InitMediaPlay();
ReadXML();
if (!m_strPictureFolder.Equals(""))
{
SearchImage(m_strPictureFolder);
imageListBox.ItemsSource = m_picureInfoList;
if (m_picureInfoList.Count > 0)
{
m_picutureInfoViewModel.ImagePath = m_picureInfoList[0].ImagePath;
ShowImage(m_picutureInfoViewModel.ImagePath);
}
}
if (!m_strMusicFolder.Equals(""))
{
SearchMusic(m_strMusicFolder);
musicListBox.ItemsSource = m_musicList;
}
}
/** 图片信息 **/
private List m_picureInfoList = null;
private int m_ImageIndex = 0;
private string m_strPictureFolder = "";
private PicutureInfo m_picutureInfoViewModel = new PicutureInfo();
private bool m_bPicturePlay = false;
private bool m_bOnePictureFinished = false;
/** 音乐信息 **/
private MediaElement mediaPlayer = new MediaElement();
private List m_musicList = null;
private int m_MusicIndex = 0;
private string m_strMusicFolder = "";
/*** 用于显示图片动画 ***/
private int m_iRectSize = 100; //设置矩形的大小
private int m_iCurRectSize = 0; //矩形的初始宽度
private PathGeometry m_pathGeometry = new PathGeometry();
private DispatcherTimer m_imageRectTimer = new DispatcherTimer();
private int m_imageWidth = 0;
private int m_imageHeight = 0;
private DispatcherTimer m_timer = new DispatcherTimer();//创建定时器对象
void Timer_Tick(object sender, EventArgs e)
{
if (m_bOnePictureFinished)
{
if (m_ImageIndex + 1 >= m_picureInfoList.Count)
{
m_ImageIndex = 0;
}
else
{
m_ImageIndex++;
}
m_picutureInfoViewModel.ImagePath = m_picureInfoList[m_ImageIndex].ImagePath;
ShowImage(m_picutureInfoViewModel.ImagePath);
}
}
void Timer_Init()
{
m_timer.Tick += new EventHandler(Timer_Tick); //添加事件,定时到事件
m_timer.Interval = TimeSpan.FromMilliseconds(3000);//设置定时长
}
void Timer_Stop()
{
m_timer.Stop();
}
void Timer_Start()
{
m_timer.Start();
}
private void InitMediaPlay()
{
mediaPlayer.LoadedBehavior = MediaState.Manual; //设置为手动控制
mediaPlayer.UnloadedBehavior = MediaState.Manual;
mediaPlayer.IsHitTestVisible = true;
mediaPlayer.MediaEnded += new RoutedEventHandler(me_MediaEnded);
gridCtr.Children.Add(mediaPlayer);
}
private void me_MediaEnded(object sender, RoutedEventArgs e)
{
StopMusic();
if (m_MusicIndex + 1 >= m_musicList.Count)
{
m_MusicIndex = 0;
}
else
{
m_MusicIndex++;
}
PlayMusic();
}
private void PlayMusic()
{
Uri uri = new Uri(m_musicList[m_MusicIndex].MusicPath, UriKind.Relative);
mediaPlayer.Source = uri;
musicListBox.Focus();
musicListBox.SelectedIndex = m_MusicIndex;
mediaPlayer.Play();
}
private void StopMusic()
{
mediaPlayer.Stop();
}
private void SearchImage(string path)
{
m_picureInfoList.Clear();
DirectoryInfo folder = new DirectoryInfo(path);
foreach (FileInfo file in folder.GetFiles("*.*"))
{
if (IsPhotoFile(file.Extension))
{
PicutureInfo Info = new PicutureInfo();
Info.ImagePath = file.FullName;
m_picureInfoList.Add(Info);
}
}
}
private void SearchMusic(string path)
{
m_musicList.Clear();
DirectoryInfo folder = new DirectoryInfo(path);
foreach (FileInfo file in folder.GetFiles("*.*"))
{
if (IsMusicFile(file.Extension))
{
MusicInfo Info = new MusicInfo();
Info.MusicPath = file.FullName;
Info.MusicTitle = file.Name;
m_musicList.Add(Info);
}
}
}
private bool IsMusicFile(string extension)
{
string[] exArray = { ".MP3", ".WAVE", ".WMA", ".MIDI" };
foreach (string strExe in exArray)
{
if (extension.ToUpper().Equals(strExe))
{
return true;
}
}
return false;
}
private bool IsPhotoFile(string extension)
{
string[] exArray = { ".PNG", ".JPG", ".BMP", ".GIF", ".JPEG"};
foreach(string strExe in exArray)
{
if (extension.ToUpper().Equals(strExe))
{
return true;
}
}
return false;
}
private void bntSelectPhoto_Click(object sender, RoutedEventArgs e)
{
FolderBrowserDialog dialog = new FolderBrowserDialog();
dialog.Description = "请选择照片目录路径";
if (dialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
string foldPath = dialog.SelectedPath;
m_strPictureFolder = foldPath;
SearchImage(foldPath);
imageListBox.ItemsSource = m_picureInfoList;
if (m_picureInfoList.Count > 0)
{
m_picutureInfoViewModel.ImagePath = m_picureInfoList[0].ImagePath;
ShowImage(m_picutureInfoViewModel.ImagePath);
}
}
}
private void bntSelectMusic_Click(object sender, RoutedEventArgs e)
{
FolderBrowserDialog dialog = new FolderBrowserDialog();
dialog.Description = "请选择音乐目录路径";
if (dialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
string foldPath = dialog.SelectedPath;
m_strMusicFolder = foldPath;
SearchMusic(foldPath);
musicListBox.ItemsSource = m_musicList;
}
}
private void bntViewPhotos_Click(object sender, RoutedEventArgs e)
{
if (m_musicList.Count > 0)
{
PlayMusic();
}
if (m_picureInfoList.Count> 0)
{
Timer_Start();
m_bPicturePlay = true;
}
}
private void InitShowImageTimer()
{
m_imageRectTimer.Interval = TimeSpan.FromMilliseconds(1);
m_imageRectTimer.Tick += new EventHandler(ImageRectTimer_Tick);
}
private void ShowImage(string strImagePath)
{
Bitmap pic = new Bitmap(m_picutureInfoViewModel.ImagePath);
m_imageWidth = pic.Size.Width; // 图片的宽度
m_imageHeight = pic.Size.Height; // 图片的高度
// m_imageRectTimer.Stop();
m_bOnePictureFinished = false;
if (m_pathGeometry != null)
{
m_pathGeometry.Clear();
}
m_imageRectTimer.Start();
}
private void ImageRectTimer_Tick(object sender, EventArgs e)
{
if (m_iCurRectSize 0)
{
StopMusic();
}
SaveXML();
}
private void SaveXML()
{
try
{
string strPath = string.Format("{0}\\PictureMagic.xml", System.Windows.Forms.Application.StartupPath);
XDocument xDoc = new XDocument();
XElement xRoot = new XElement("Settings");
xDoc.Add(xRoot);
XElement pf = new XElement("PictureFolder");
pf.Value = m_strPictureFolder;
XElement mf = new XElement("MusicFolder");
mf.Value = m_strMusicFolder;
xRoot.Add(pf, mf);
xDoc.Save(strPath);
}catch(Exception)
{
}
}
private void ReadXML()
{
string strPath = string.Format("{0}\\PictureMagic.xml", System.Windows.Forms.Application.StartupPath);
try
{
XDocument xDoc = XDocument.Load(strPath);
XElement pf = xDoc.Root.Element("PictureFolder");
m_strPictureFolder = pf.Value;
XElement mf = xDoc.Root.Element("MusicFolder");
m_strMusicFolder = mf.Value;
}
catch (Exception)
{
}
}
private void TextBlock_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
if (e.ClickCount>= 2)
{
int selectIndex = musicListBox.SelectedIndex;
if (selectIndex >= 0)
{
StopMusic();
m_MusicIndex = selectIndex;
PlayMusic();
if (!m_bPicturePlay)
{
Timer_Start();
m_bPicturePlay = true;
}
}
}
}
}
}
WPF 制作电子相册浏览器
标签:比较 linq int cli 手动 lin ret blog osi
原文地址:https://www.cnblogs.com/lonelyxmas/p/9706998.html
评论