标签:item 选中 rtl name set protected tip bool collect
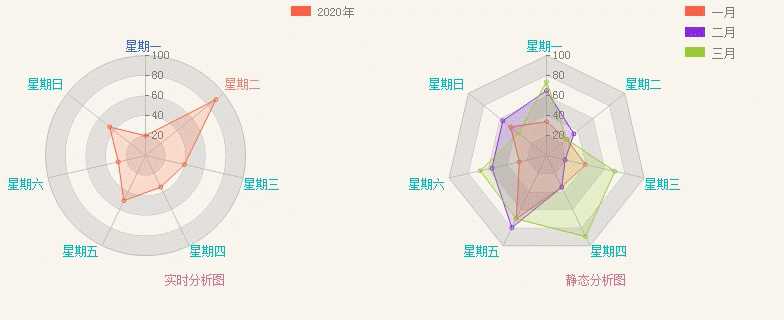
///
/// 雷达分析图控件
///
[ToolboxItem(true)]
[DefaultProperty("ChartLineItems")]
[Description("雷达分析图控件")]
public partial class RadarChartExt : Control
{
#region
private ChartTypes chartType = ChartTypes.Circle;
///
/// 雷达分析图显示类型
///
[DefaultValue(ChartTypes.Circle)]
[Description("雷达分析图显示类型")]
public ChartTypes ChartType
{
get { return this.chartType; }
set
{
if (this.chartType == value)
return;
this.chartType = value;
this.Invalidate();
}
}
private ChartAnchors chartAnchor = ChartAnchors.Left;
///
/// 分析图布局
///
[DefaultValue(ChartAnchors.Left)]
[Description("分析图布局")]
public ChartAnchors ChartAnchor
{
get { return this.chartAnchor; }
set
{
if (this.chartAnchor == value)
return;
this.chartAnchor = value;
this.InitializeRectangle();
this.Invalidate();
}
}
private DescriptionAnchors descriptionAnchor = DescriptionAnchors.Bottom;
///
/// 描述布局
///
[DefaultValue(DescriptionAnchors.Bottom)]
[Description("描述布局")]
public DescriptionAnchors DescriptionAnchor
{
get { return this.descriptionAnchor; }
set
{
if (this.descriptionAnchor == value)
return;
this.descriptionAnchor = value;
this.InitializeRectangle();
this.Invalidate();
}
}
private Size chartSize = new Size(200, 200);
///
/// 分析图Size
///
[DefaultValue(typeof(Size), "200, 200")]
[Description("分析图Size")]
public Size ChartSize
{
get { return this.chartSize; }
set
{
if (this.chartSize == value || value.Width 2 || value.Height 2)
return;
this.chartSize = value;
this.InitializeRectangle();
this.Invalidate();
}
}
private int loopCount = 5;
///
/// 环形数量
///
[DefaultValue(5)]
[Description("环形数量")]
public int LoopCount
{
get { return this.loopCount; }
set
{
if (this.loopCount == value || value 1)
return;
this.loopCount = value;
this.InitializeLoop();
this.InitializeRectangle();
this.Invalidate();
}
}
private float loopInterval = 20f;
///
/// 环形间隔值
///
[DefaultValue(20f)]
[Description("环形间隔值")]
public float LoopInterval
{
get { return this.loopInterval; }
set
{
if (this.loopInterval == value || value 0f)
return;
this.loopInterval = value;
this.InitializeRectangle();
this.Invalidate();
}
}
private int loopBorderthickness = 1;
///
/// 环形线厚度
///
[DefaultValue(1)]
[Description("环形线厚度")]
public int LoopBorderthickness
{
get { return this.loopBorderthickness; }
set
{
if (this.loopBorderthickness == value || this.loopBorderthickness 1)
return;
this.loopBorderthickness = value;
this.Invalidate();
}
}
private Color loopBorderColor = Color.FromArgb(224, 224, 224);
///
/// 环形线颜色
///
[DefaultValue(typeof(Color), "224, 224, 224")]
[Description("环形线颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color LoopBorderColor
{
get { return this.loopBorderColor; }
set
{
if (this.loopBorderColor == value)
return;
this.loopBorderColor = value;
this.Invalidate();
}
}
private Color loopOddBackColor = Color.FromArgb(62, 224, 224, 224);
///
/// 奇数环形背景颜色
///
[DefaultValue(typeof(Color), "62, 224, 224, 224")]
[Description("奇数环形背景颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color LoopOddBackColor
{
get { return this.loopOddBackColor; }
set
{
if (this.loopOddBackColor == value)
return;
this.loopOddBackColor = value;
this.Invalidate();
}
}
private Color loopEvenBackColor = Color.FromArgb(255, 255, 255);
///
/// 偶数环形背景颜色
///
[DefaultValue(typeof(Color), "255, 255, 255")]
[Description("偶数环形背景颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color LoopEvenBackColor
{
get { return this.loopEvenBackColor; }
set
{
if (this.loopEvenBackColor == value)
return;
this.loopEvenBackColor = value;
this.Invalidate();
}
}
private Color loopScaleTextColor = Color.FromArgb(128, 128, 128);
///
/// 环形刻度文本颜色
///
[DefaultValue(typeof(Color), "128, 128, 128")]
[Description("环形刻度文本颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color LoopScaleTextColor
{
get { return this.loopScaleTextColor; }
set
{
if (this.loopScaleTextColor == value)
return;
this.loopScaleTextColor = value;
this.Invalidate();
}
}
private Color loopLineTextColor = Color.FromArgb(0, 192, 192);
///
/// 环形选项文本颜色
///
[DefaultValue(typeof(Color), "0, 192, 192")]
[Description("环形选项文本颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color LoopLineTextColor
{
get { return this.loopLineTextColor; }
set
{
if (this.loopLineTextColor == value)
return;
this.loopLineTextColor = value;
this.Invalidate();
}
}
private bool loopLineMaxValueShow = false;
///
/// 动画中是否显示环形选项最大值高亮背景颜色
///
[DefaultValue(false)]
[Description("动画中是否显示环形选项最大值高亮背景颜色")]
public bool LoopLineMaxValueShow
{
get { return this.loopLineMaxValueShow; }
set
{
if (this.loopLineMaxValueShow == value)
return;
this.loopLineMaxValueShow = value;
this.Invalidate();
}
}
private Color loopLineMaxValueTextColor = Color.FromArgb(255, 128, 128);
///
/// 环形选项最大值高亮背景颜色
///
[DefaultValue(typeof(Color), "255, 128, 128")]
[Description("环形选项最大值高亮背景颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color LoopLineMaxValueTextColor
{
get { return this.loopLineMaxValueTextColor; }
set
{
if (this.loopLineMaxValueTextColor == value)
return;
this.loopLineMaxValueTextColor = value;
this.Invalidate();
}
}
private bool loopLineMinValueShow = false;
///
/// 动画中是否显示环形选项最小值高亮背景颜色
///
[DefaultValue(false)]
[Description("动画中是否显示环形选项最小值高亮背景颜色")]
public bool LoopLineMinValueShow
{
get { return this.loopLineMinValueShow; }
set
{
if (this.loopLineMinValueShow == value)
return;
this.loopLineMinValueShow = value;
this.Invalidate();
}
}
private Color loopLineMinValueTextColor = Color.FromArgb(65, 105, 225);
///
/// 环形选项最小值高亮背景颜色
///
[DefaultValue(typeof(Color), "65, 105, 225")]
[Description("环形选项最小值高亮背景颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color LoopLineMinValueTextColor
{
get { return this.loopLineMinValueTextColor; }
set
{
if (this.loopLineMinValueTextColor == value)
return;
this.loopLineMinValueTextColor = value;
this.Invalidate();
}
}
private bool optionAreaShow = true;
///
/// 是否显示选项列表
///
[DefaultValue(true)]
[Description("是否显示选项列表")]
public bool OptionAreaShow
{
get { return this.optionAreaShow; }
set
{
if (this.optionAreaShow == value)
return;
this.optionAreaShow = value;
this.InitializeRectangle();
this.Invalidate();
}
}
private int optionAreaWidth = 40;
///
/// 选项列表区域宽度
///
[DefaultValue(40)]
[Description("选项列表区域宽度")]
public int OptionAreaWidth
{
get { return this.optionAreaWidth; }
set
{
if (this.optionAreaWidth == value || this.optionAreaWidth 0)
return;
this.optionAreaWidth = value;
this.InitializeRectangle();
this.Invalidate();
}
}
private int descriptionAreaHeight = 30;
///
/// 文本区域高度
///
[DefaultValue(30)]
[Description("文本区域高度")]
public int DescriptionAreaHeight
{
get { return this.descriptionAreaHeight; }
set
{
if (this.descriptionAreaHeight == value || this.descriptionAreaHeight 0)
return;
this.descriptionAreaHeight = value;
this.InitializeRectangle();
this.Invalidate();
}
}
private Color optionTextColor = Color.FromArgb(128, 128, 128);
///
/// 选项文本颜色
///
[DefaultValue(typeof(Color), "128, 128, 128")]
[Description("选项文本颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color OptionTextColor
{
get { return this.optionTextColor; }
set
{
if (this.optionTextColor == value)
return;
this.optionTextColor = value;
this.Invalidate();
}
}
private Color optionTipTextColor = Color.FromArgb(255, 255, 255);
///
/// 选项提示文本颜色
///
[DefaultValue(typeof(Color), "255, 255, 255")]
[Description("选项提示文本颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color OptionTipTextColor
{
get { return this.optionTipTextColor; }
set
{
if (this.optionTipTextColor == value)
return;
this.optionTipTextColor = value;
this.Invalidate();
}
}
private string title = "";
///
/// 标题
///
[DefaultValue("")]
[Description("标题")]
public string Title
{
get { return this.title; }
set
{
if (this.title == value)
return;
this.title = value;
this.Invalidate();
}
}
private Color titleColor = Color.FromArgb(219, 112, 147);
///
/// 标题颜色
///
[DefaultValue(typeof(Color), "219, 112, 147")]
[Description("标题颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color TitleColor
{
get { return this.titleColor; }
set
{
if (this.titleColor == value)
return;
this.titleColor = value;
this.Invalidate();
}
}
private ChartLoopCollection chartLoopCollection;
///
/// 分析图环形配置集合
///
[Browsable(false)]
[Description("分析图环形配置集合")]
public ChartLoopCollection ChartLoopItems
{
get
{
if (this.chartLoopCollection == null)
this.chartLoopCollection = new ChartLoopCollection(this);
return this.chartLoopCollection;
}
}
private ChartLineCollection chartLineCollection;
///
/// 分析图角度线配置集合
///
[Description("分析图角度线配置集合")]
[DesignerSerializationVisibility(DesignerSerializationVisibility.Content)]
public ChartLineCollection ChartLineItems
{
get
{
if (this.chartLineCollection == null)
this.chartLineCollection = new ChartLineCollection(this);
return this.chartLineCollection;
}
}
private ChartItemCollection chartItemOptionCollection;
///
/// 分析图选项数据集合
///
[Browsable(false)]
[Description("分析图选项数据集合")]
[DesignerSerializationVisibility(DesignerSerializationVisibility.Content)]
public ChartItemCollection ChartItemItems
{
get
{
if (this.chartItemOptionCollection == null)
this.chartItemOptionCollection = new ChartItemCollection(this);
return this.chartItemOptionCollection;
}
}
protected override Size DefaultSize
{
get
{
return new Size(150, 150); ;
}
}
public override string Text
{
get
{
return base.Text;
}
set
{
if (base.Text == value)
return;
base.Text = value;
this.Invalidate();
}
}
protected override Padding DefaultPadding
{
get
{
return new Padding(10);
}
}
public new Padding Padding
{
get
{
return base.Padding;
}
set
{
if (this.Padding == value)
return;
this.Padding = value;
this.InitializeRectangle();
this.Invalidate();
}
}
///
/// 是否启用动画变换
///
private bool isAnimation = false;
///
/// 雷达分析图信息
///
private ChartRectangle ChartMainRect;
///
/// 分析图选项状态
///
private ChartItemMoveStatuss ChartItemMoveStatus = ChartItemMoveStatuss.Normal;
///
/// 默认开始角度
///
private float DefaultAngle = 270f;
///
/// 选中环形对角线
///
private ItemLine SelectItemLine = null;
///
/// 动画播放定时器
///
private AnimationTimer animationTimer;
#endregion
public RadarChartExt()
{
SetStyle(ControlStyles.UserPaint, true);
SetStyle(ControlStyles.AllPaintingInWmPaint, true);
SetStyle(ControlStyles.OptimizedDoubleBuffer, true);
SetStyle(ControlStyles.ResizeRedraw, true);
SetStyle(ControlStyles.SupportsTransparentBackColor, true);
InitializeComponent();
this.ChartMainRect = new ChartRectangle();
this.InitializeLoop();
this.InitializeRectangle();
}
#region 重写
protected override void OnPaint(System.Windows.Forms.PaintEventArgs e)
{
base.OnPaint(e);
if (this.ChartLineItems.Count 3)
return;
Graphics g = e.Graphics;
SmoothingMode sm = g.SmoothingMode;
g.SmoothingMode = SmoothingMode.AntiAlias;
GraphicsPath loop_gp = new GraphicsPath();
#region 环形
Pen loopBorder_pen = new Pen(this.LoopBorderColor, this.LoopBorderthickness);
SolidBrush loopoddback_sb = new SolidBrush(this.LoopOddBackColor);
SolidBrush loopevenback_sb = new SolidBrush(this.LoopEvenBackColor);
SolidBrush looplinetext_sb = new SolidBrush(this.LoopLineTextColor);
SolidBrush looplinemaxvaluetext_sb = this.isAnimation && this.LoopLineMaxValueShow ? new SolidBrush(this.LoopLineMaxValueTextColor) : null;
SolidBrush looplineminvaluetext_sb = this.isAnimation && this.LoopLineMinValueShow ? new SolidBrush(this.LoopLineMinValueTextColor) : null;
for (int i = 0; i this.ChartLoopItems.Count; i++)
{
if (this.ChartLoopItems[i].DataPoints.Length 3)
continue;
if (this.ChartType == ChartTypes.Rhombus)
{
loop_gp.Reset();
loop_gp.AddPolygon(this.ChartLoopItems[i].DataPoints);
loop_gp.CloseFigure();
g.DrawPath(loopBorder_pen, loop_gp);
loop_gp.Reset();
loop_gp.AddPolygon(this.ChartLoopItems[i].DataPoints);
if (i > 0)
{
loop_gp.StartFigure();
loop_gp.AddPolygon(this.ChartLoopItems[i - 1].DataPoints);
}
g.FillPath((i % 2 == 0) ? loopoddback_sb : loopevenback_sb, loop_gp);
}
else
{
loop_gp.Reset();
loop_gp.AddEllipse(new RectangleF(this.ChartLoopItems[i].LoopCenter.X - this.ChartLoopItems[i].LoopRadius, this.ChartLoopItems[i].LoopCenter.Y - this.ChartLoopItems[i].LoopRadius, this.ChartLoopItems[i].LoopRadius * 2, this.ChartLoopItems[i].LoopRadius * 2));
g.DrawPath(loopBorder_pen, loop_gp);
loop_gp.Reset();
loop_gp.AddEllipse(new RectangleF(this.ChartLoopItems[i].LoopCenter.X - this.ChartLoopItems[i].LoopRadius, this.ChartLoopItems[i].LoopCenter.Y - this.ChartLoopItems[i].LoopRadius, this.ChartLoopItems[i].LoopRadius * 2, this.ChartLoopItems[i].LoopRadius * 2));
if (i > 0)
{
loop_gp.StartFigure();
loop_gp.AddEllipse(new RectangleF(this.ChartLoopItems[i - 1].LoopCenter.X - this.ChartLoopItems[i - 1].LoopRadius, this.ChartLoopItems[i - 1].LoopCenter.Y - this.ChartLoopItems[i - 1].LoopRadius, this.ChartLoopItems[i - 1].LoopRadius * 2, this.ChartLoopItems[i - 1].LoopRadius * 2));
}
g.FillPath((i % 2 == 0) ? loopoddback_sb : loopevenback_sb, loop_gp);
}
}
#endregion
#region 角度线
float maxValue = 0f;
float minValue = 0f;
Listint> maxIndexList = new Listint>();
Listint> minIndexList = new Listint>();
if (this.LoopLineMaxValueShow && this.ChartItemItems.Count > 0)
{
for (int i = 0; i this.ChartItemItems[0].ItemDataTargrt.Length; i++)
{
if (i == 0)
{
maxValue = this.ChartItemItems[0].ItemDataTargrt[0];
}
if (this.ChartItemItems[0].ItemDataTargrt[i] > maxValue)
{
maxValue = this.ChartItemItems[0].ItemDataTargrt[i];
maxIndexList.Clear();
maxIndexList.Add(i);
}
else if (this.ChartItemItems[0].ItemDataTargrt[i] == maxValue)
{
maxIndexList.Add(i);
}
}
}
if (this.LoopLineMinValueShow && this.ChartItemItems.Count > 0)
{
for (int i = 0; i this.ChartItemItems[0].ItemDataTargrt.Length; i++)
{
if (i == 0)
{
minValue = this.ChartItemItems[0].ItemDataTargrt[0];
}
if (this.ChartItemItems[0].ItemDataTargrt[i] minValue)
{
minValue = this.ChartItemItems[0].ItemDataTargrt[i];
minIndexList.Clear();
minIndexList.Add(i);
}
else if (this.ChartItemItems[0].ItemDataTargrt[i] == minValue)
{
minIndexList.Add(i);
}
}
}
for (int i = 0; i this.ChartLineItems.Count; i++)
{
string loopline_txt = this.ChartLineItems[i].LineText;
SizeF loopline_textsize = g.MeasureString(loopline_txt, this.Font);
PointF loopline_txt_point = this.ChartLineItems[i].LineEndPoint;
if (this.ChartLineItems[i].LineAngle == 0)
{
loopline_txt_point.Y = loopline_txt_point.Y - loopline_textsize.Height / 2;
}
else if (this.ChartLineItems[i].LineAngle 90)
{
}
else if (this.ChartLineItems[i].LineAngle == 90)
{
loopline_txt_point.X = loopline_txt_point.X - loopline_textsize.Width / 2;
}
else if (this.ChartLineItems[i].LineAngle 180)
{
loopline_txt_point.X = loopline_txt_point.X - loopline_textsize.Width;
}
else if (this.ChartLineItems[i].LineAngle == 180)
{
loopline_txt_point.Y = loopline_txt_point.Y - loopline_textsize.Height / 2;
loopline_txt_point.X = loopline_txt_point.X - loopline_textsize.Width;
}
else if (this.ChartLineItems[i].LineAngle 270)
{
loopline_txt_point.Y = loopline_txt_point.Y - loopline_textsize.Height;
loopline_txt_point.X = loopline_txt_point.X - loopline_textsize.Width;
}
else if (this.ChartLineItems[i].LineAngle == 270)
{
loopline_txt_point.Y = loopline_txt_point.Y - loopline_textsize.Height;
loopline_txt_point.X = loopline_txt_point.X - loopline_textsize.Width / 2;
}
else if (this.ChartLineItems[i].LineAngle 360)
{
loopline_txt_point.Y = loopline_txt_point.Y - loopline_textsize.Height;
}
SolidBrush sb = looplinetext_sb;
if (this.isAnimation)
{
if (maxIndexList.IndexOf(i) != -1)
{
sb = looplinemaxvaluetext_sb;
}
if (minIndexList.IndexOf(i) != -1)
{
sb = looplineminvaluetext_sb;
}
}
g.DrawString(loopline_txt, this.Font, sb, loopline_txt_point);
}
for (int j = 0; j this.ChartLineItems.Count; j++)
{
g.DrawLine(loopBorder_pen, this.ChartLineItems[j].LoopCenter, this.ChartLineItems[j].LineEndPoint);
}
#endregion
loop_gp.Dispose();
loopBorder_pen.Dispose();
loopoddback_sb.Dispose();
loopevenback_sb.Dispose();
looplinetext_sb.Dispose();
if (looplinemaxvaluetext_sb != null)
looplinemaxvaluetext_sb.Dispose();
if (looplineminvaluetext_sb != null)
looplineminvaluetext_sb.Dispose();
#region 数据
SolidBrush databack_sb = new SolidBrush(Color.White);
if (this.ChartItemItems.Count > 0)
{
GraphicsPath databack_gp = new GraphicsPath();
Pen databorder_pen = new Pen(Color.White, 1);
Pen datadot_pen = new Pen(Color.White, 1);
for (int i = 0; i this.ChartItemItems.Count; i++)
{
if (this.ChartItemItems[i].ItemDataPoints.Length 2)
continue;
databack_gp.Reset();
databack_gp.AddPolygon(this.ChartItemItems[i].ItemDataPoints);
databack_gp.CloseFigure();
databack_sb.Color = Color.FromArgb(50, this.ChartItemItems[i].ItemBackColor);
g.FillPath(databack_sb, databack_gp);
databorder_pen.Color = this.ChartItemItems[i].ItemBackColor;
g.DrawPath(databorder_pen, databack_gp);
datadot_pen.Color = this.ChartItemItems[i].ItemBackColor;
for (int j = 0; j this.ChartItemItems[i].ItemDataPoints.Length; j++)
{
g.DrawEllipse(datadot_pen, this.ChartItemItems[i].ItemDataRectF[j]);
}
}
databack_gp.Dispose();
databorder_pen.Dispose();
datadot_pen.Dispose();
}
#endregion
g.SmoothingMode = sm;
#region 数据选项
if (this.OptionAreaShow)
{
float desc_padding = 5;
float descitem_height = 0;
SolidBrush descitem_sb = new SolidBrush(Color.White);
SolidBrush desctext_sb = new SolidBrush(this.OptionTextColor);
for (int i = 0; i this.ChartItemItems.Count; i++)
{
descitem_sb.Color = this.ChartItemItems[i].ItemBackColor;
SizeF desc_size = g.MeasureString(&l