标签:注意 tac rem cte input time value 列表 hashset
概述
Redis【REmote DIctionary Server】作为当前比较流行的NoSql数据库,以其高性能,高可用的的特点,应用越来越广泛,深得各大企业和开发人员的青睐。 本文主要以一个简单的小例子,简述ServiceStack.Redis动态库在Redis方面的相关应用,仅供学习分享使用,如有不足之处,还请指正。
开发环境
相关开发环境,如下所示:
- Microsoft Visual Studio Community 2019
- ServiceStack.Redis 动态库
- Redis 6.0.9 服务器端环境搭建
ServiceStack.Redis的安装
在C#开发中,主要通过NuGet包管理器,来安装ServiceStack.Redis动态库,目前版本为5.10.4,如下所示:
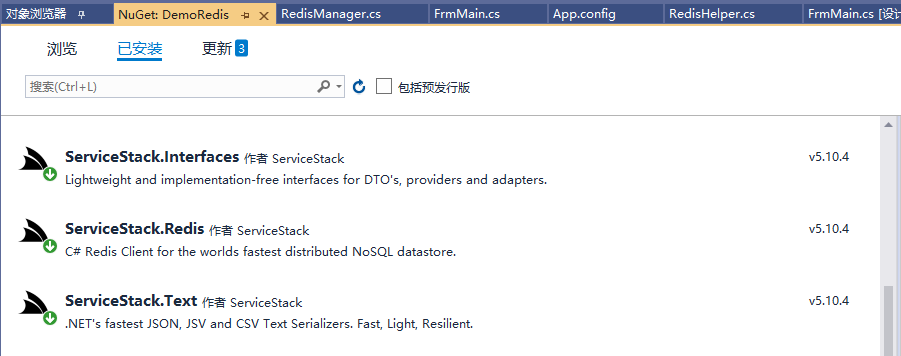
示例截图
关于C#调用Redis相关示例截图,如下所示:
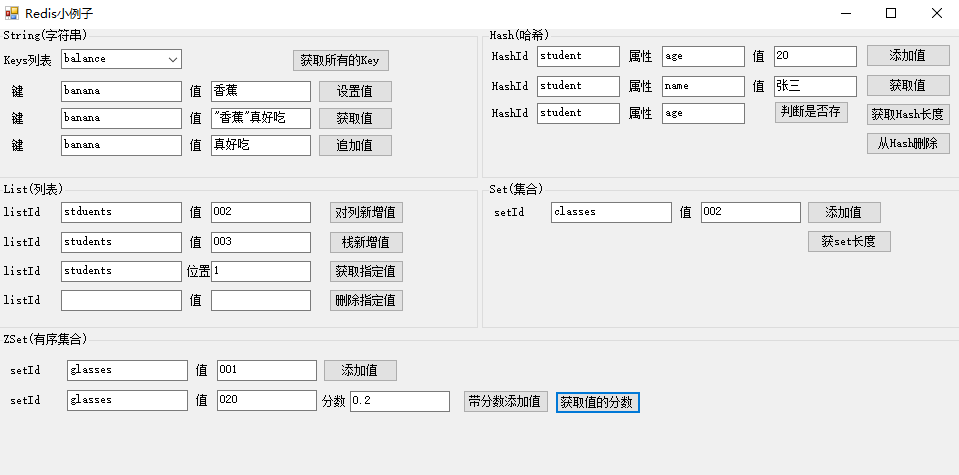
核心代码
关于Redis操作的核心代码,主要分两部分:Redis客户端管理类,Redis客户端操作类。
Redis客户端管理类,主要通过Redis客户端连接池【PooledRedisClientManager】创建客户端对象【IRedisClient】,如下所示:


1 using ServiceStack.Redis;
2 using System;
3 using System.Collections.Generic;
4 using System.Linq;
5 using System.Text;
6 using System.Threading.Tasks;
7
8 namespace DemoRedis
9 {
10 ///
11 /// Redis客户端管理类
12 ///
13 public static class RedisManager
14 {
15 private static PooledRedisClientManager clientManager;
16
17 ///
18 /// 初始化信息
19 ///
20 private static void initInfo()
21 {
22 string ipaddr = System.Configuration.ConfigurationManager.AppSettings["ipaddr"];
23 string port = System.Configuration.ConfigurationManager.AppSettings["port"];
24 string host = string.Format("{0}:{1}", ipaddr, port);
25 initInfo(new string[] { host }, new string[] { host });
26 }
27
28 ///
29 /// 初始化Redis客户端管理
30 ///
31 ///
32 ///
33 private static void initInfo(string[] readWriteHosts, string[] readOnlyHosts)
34 {
35 clientManager = new PooledRedisClientManager(readWriteHosts, readOnlyHosts, new RedisClientManagerConfig
36 {
37 MaxWritePoolSize = 5, // “写”链接池链接数
38 MaxReadPoolSize = 5, // “读”链接池链接数
39 AutoStart = true,
40 });
41 }
42
43 public static IRedisClient getRedisClient()
44 {
45 if (clientManager == null)
46 {
47 initInfo();
48 }
49 return clientManager.GetClient();
50 }
51
52 }
53
54
55 }
View Code
Redis客户端操作类,主要包括字符串(String),列表(List),哈希结构(Hash),集合(Set),有序集合(Sorted Set)等相关操作功能的封装,如下所示:


1 using ServiceStack.Redis;
2 using System;
3 using System.Collections.Generic;
4 using System.Linq;
5 using System.Text;
6 using System.Threading.Tasks;
7
8 namespace DemoRedis
9 {
10 public class RedisHelper:IDisposable
11 {
12 private IRedisClient client;
13
14 public RedisHelper()
15 {
16
17 }
18
19 public RedisHelper(IRedisClient client ) {
20 this.client = client;
21 }
22
23 #region 字符串
24
25 public Liststring> getAllKeys()
26 {
27 return this.client.GetAllKeys();
28 }
29
30 ///
31 /// 设置值
32 ///
33 ///
34 ///
35 public void setString(string key,string value)
36 {
37 this.client.Set(key, value);
38 }
39
40 ///
41 /// 设置值,带过期时间
42 ///
43 ///
44 ///
45 ///
46 public void setString(string key, string value,TimeSpan expiresIn)
47 {
48 this.client.Set(key, value, expiresIn);
49 }
50
51 ///
52 /// 是否存在
53 ///
54 ///
55 ///
56 public bool containsKey(string key)
57 {
58 return this.client.ContainsKey(key);
59 }
60
61 ///
62 /// 设置字典
63 ///
64 ///
65 public void setAll(Dictionarystring,string> dic)
66 {
67 this.client.SetAll(dic);
68 }
69
70 ///
71 /// 返回旧值,设置新值
72 ///
73 ///
74 ///
75 ///
76 public string getAndSetValue(string key,string value) {
77 return this.client.GetAndSetValue(key, value);
78 }
79
80 ///
81 /// 获取对应的值
82 ///
83 ///
84 ///
85 public string getValue(string key) {
86 return this.client.GetValue(key);
87 }
88
89 public string getRandomKey()
90 {
91 return this.client.GetRandomKey();
92 }
93
94 ///
95 /// 追加值到现有key中
96 ///
97 ///
98 ///
99 public void appendToValue(string key, string value) {
100 this.client.AppendToValue(key, value);
101 }
102
103 #endregion
104
105 #region List
106
107 ///
108 /// (队列操作)增加一个值到列表
109 ///
110 ///
111 ///
112 public void enqueueItemOnList(string listId ,string value)
113 {
114 this.client.EnqueueItemOnList(listId, value);
115 }
116
117 ///
118 /// 从队列中出一个
119 ///
120 ///
121 ///
122 public string dequeueItemFromList(string listId)
123 {
124 return this.client.DequeueItemFromList(listId);
125 }
126
127 ///
128 /// 栈,增加一个值到列表
129 ///
130 ///
131 ///
132 public void pushItemToList(string listId, string value) {
133 this.client.PushItemToList(listId, value);
134 }
135
136 ///
137 /// 栈,从当前列表中出一个值,并返回
138 ///
139 ///
140 ///
141 public string popItemFromList(string listId)
142 {
143 return this.client.PopItemFromList(listId);
144 }
145
146
147 ///
148 /// 获取某一个位置的值
149 ///
150 ///
151 ///
152 ///
153 public string getItemFromList(string listId, int index)
154 {
155 return this.client.GetItemFromList(listId, index);
156 }
157
158 ///
159 /// 设置并修改指定位置的值
160 ///
161 ///
162 ///
163 ///
164 public void setItemInList(string listId, int index,string value)
165 {
166 this.client.SetItemInList(listId, index, value);
167 }
168
169
170 ///
171 /// 获取列表所有的值
172 ///
173 ///
174 ///
175 public Liststring> getAllItemsFromList(string listId) {
176 return this.client.GetAllItemsFromList(listId);
177 }
178
179 ///
180 /// 删除所有内容
181 ///
182 ///
183 public void removeAllFromList(string listId) {
184 this.client.RemoveAllFromList(listId);
185 }
186
187 ///
188 /// 删除列表指定元素
189 ///
190 ///
191 ///
192 public void removeItemFromList(string listId, string value) {
193 this.client.RemoveItemFromList(listId, value);
194 }
195
196 ///
197 /// 获取指定列表的长度
198 ///
199 ///
200 ///
201 public long getListCount(string listId) {
202 return this.client.GetListCount(listId);
203 }
204
205 #endregion
206
207 #region Hash
208
209 ///
210 /// 设置Hash的值
211 ///
212 ///
213 ///
214 ///
215 ///
216 public bool setEntryInHash(string hashId, string key, string value)
217 {
218 return this.client.SetEntryInHash(hashId, key, value);
219 }
220
221 ///
222 /// 获取Hash中的值
223 ///
224 ///
225 ///
226 ///
227 public string getValueFromHash(string hashId, string key)
228 {
229 return this.client.GetValueFromHash(hashId, key);
230 }
231
232 ///
233 /// 获取Hash列表中的所有内容
234 ///
235 ///
236 public Dictionarystring,string> getAllEntriesFromHash(string hashId)
237 {
238 return this.client.GetAllEntriesFromHash(hashId);
239 }
240
241 ///
242 /// 判断Hash是否存在指定的键
243 ///
244 ///
245 ///
246 ///
247 public bool hashContainsEntry(string hashId, string key)
248 {
249 return this.client.HashContainsEntry(hashId, key);
250 }
251
252 ///
253 /// 设置多个值到Hash
254 ///
255 ///
256 ///
257 public void setRangeInHash(string hashId,Dictionarystring,string> keyValuePairs) {
258 this.client.SetRangeInHash(hashId, keyValuePairs);
259 }
260
261 ///
262 /// 获取Hash列表的长度
263 ///
264 ///
265 ///
266 public long getHashCount(string hashId) {
267 return this.client.GetHashCount(hashId);
268 }
269
270 ///
271 /// 删除某一个值
272 ///
273 ///
274 ///
275 ///
276 public bool removeEntryFromHash(string hashId,string key) {
277 return this.client.RemoveEntryFromHash(hashId, key);
278 }
279
280 #endregion
281
282 #region Set
283
284 ///
285 /// 从Set中获取随机值
286 ///
287 ///
288 ///
289 public string getRandomItemFromSet(string setId)
290 {
291 return this.client.GetRandomItemFromSet(setId);
292 }
293
294 ///
295 /// 获取所有的值
296 ///
297 ///
298 ///
299 public HashSetstring> getAllItemsFromSet(string setId)
300 {
301 return this.client.GetAllItemsFromSet(setId);
302 }
303
304 ///
305 /// 获取set的长度
306 ///
307 ///
308 ///
309 public long getSetCount(string setId)
310 {
311 return this.client.GetSetCount(setId);
312 }
313
314 ///
315 /// 删除某一项
316 ///
317 ///
318 ///
319 public void removeItemFromSet(string setId,string item) {
320 this.client.RemoveItemFromSet(setId, item);
321 }
322
323 ///
324 /// 新增内容
325 ///
326 ///
327 ///
328 public void addItemToSet(string setId, string item) {
329 this.client.AddItemToSet(setId, item);
330 }
331
332 ///
333 /// 增加列表到Set
334 ///
335 ///
336 ///
337 public void addRangeToSet(string setId,Liststring> items) {
338 this.client.AddRangeToSet(setId, items);
339 }
340
341 #endregion
342
343 #region zset
344
345 ///
346 /// 添加元素到排序集合
347 ///
348 ///
349 ///
350 ///
351 public bool addItemToSortedSet(string setId,string value) {
352 return this.client.AddItemToSortedSet(setId, value);
353 }
354
355 ///
356 /// 添加元素到排序集合
357 ///
358 ///
359 ///
360 ///
361 ///
362 public bool addItemToSortedSet(string setId, string value,double score)
363 {
364 return this.client.AddItemToSortedSet(setId, value,score);
365 }
366
367 ///
368 /// 增加列表到排序集合
369 ///
370 ///
371 ///
372 ///
373 ///
374 public bool addRangeToSortedSet(string setId,Liststring> values, double score) {
375 return this.client.AddRangeToSortedSet(setId, values, score);
376 }
377
378 ///
379 /// 增加列表到排序集合
380 ///
381 ///
382 ///
383 ///
384 ///
385 public bool addRangeToSortedSet(string setId, Liststring> values, long score)
386 {
387 return this.client.AddRangeToSortedSet(setId, values, score);
388 }
389
390 ///
391 /// 获取所有的集合内容
392 ///
393 ///
394 ///
395 public Liststring> getAllItemsFromSortedSet(string setId) {
396 return this.client.GetAllItemsFromSortedSet(setId);
397 }
398
399 ///
400 /// 倒序获取所有的内容
401 ///
402 ///
403 ///
404 public Liststring> getAllItemsFromSortedSetDesc(string setId) {
405 return this.client.GetAllItemsFromSortedSetDesc(setId);
406 }
407
408 ///
409 /// 带分数一起取出
410 ///
411 ///
412 ///
413 public IDictionarystring, double> getAllWithScoresFromSortedSet(string setId)
414 {
415 return this.client.GetAllWithScoresFromSortedSet(setId);
416 }
417
418 ///
419 /// 获取对应值的位置
420 ///
421 ///
422 ///
423 ///
424 public long getItemIndexInSortedSet(string setId,string value)
425 {
426 return this.client.GetItemIndexInSortedSet(setId, value);
427 }
428
429 ///
430 /// 倒序的位置
431 ///
432 ///
433 ///
434 ///
435 public long getItemIndexInSortedSetDesc(string setId, string value) {
436 return this.client.GetItemIndexInSortedSetDesc(setId, value);
437 }
438
439 ///
440 /// 获取对应元素的分数
441 ///
442 ///
443 ///
444 ///
445 public double getItemScoreInSortedSet(string setId, string value) {
446 return this.client.GetItemScoreInSortedSet(setId, value);
447 }
448
449 ///
450 ///
451 ///
452 ///
453 ///
454 ///
455 ///
456 public Liststring> getRangeFromSortedSet(string setId, int fromRank, int toRank) {
457 return this.client.GetRangeFromSortedSet(setId, fromRank, toRank);
458 }
459
460 public IDictionarystring, double> getRangeWithScoresFromSortedSet(string setId, int fromRank, int toRank) {
461 return this.client.GetRangeWithScoresFromSortedSet(setId, fromRank, toRank);
462 }
463
464 public void Dispose()
465 {
466 if(this.client != null)
467 {
468 this.client.Dispose();
469 }
470 }
471 #endregion
472 }
473 }
View Code
关于前端调用功能,主要是各个按钮对应的事件,如下所示:


1 using System;
2 using System.Collections.Generic;
3 using System.ComponentModel;
4 using System.Data;
5 using System.Drawing;
6 using System.Linq;
7 using System.Text;
8 using System.Threading.Tasks;
9 using System.Windows.Forms;
10
11 namespace DemoRedis
12 {
13 public partial class FrmMain : Form
14 {
15
16 private RedisHelper redisHelper;
17
18 public FrmMain()
19 {
20 InitializeComponent();
21 }
22
23 ///
24 /// 加载
25 ///
26 ///
27 ///
28 private void FrmMain_Load(object sender, EventArgs e)
29 {
30 this.redisHelper = new RedisHelper(RedisManager.getRedisClient());
31 }
32
33 ///
34 /// 获取所有的Key
35 ///
36 ///
37 ///
38 private void btnAllKey_Click(object sender, EventArgs e)
39 {
40 Liststring> keys = this.redisHelper.getAllKeys();
41 this.combKeys.Items.AddRange(keys.ToArray());
42 this.combKeys.SelectedIndex = 0;
43 }
44
45 private void btnSetString_Click(object sen