标签:creates itme define validate size spl ror rectangle created
5.1 GDI的结构
图形设备接口(GDI:Graphics Device Interface)是Windows的子系统,它负责在视讯显示器和打印机上显示图形。
5.2 设备环境
5.2.1 获取设备环境句柄
最常用的取得并释放设备内容句柄的方法是,在处理WM_PAINT消息时,使用BeginPaint和EndPaint呼叫:
hdc = BeginPaint (hwnd, &ps) ;
其它行程序
EndPaint (hwnd, &ps) ;
Windows程序还可以在处理非WM_PAINT消息时取得设备内容句柄:
hdc = GetDC (hwnd) ;
其它行程序
ReleaseDC (hwnd, hdc) ;
Windows程序还可以取得适用于整个窗口(而不仅限于窗口的显示区域)的设备内容句柄:
hdc = GetWindowDC (hwnd) ;
其它行程序
ReleaseDC (hwnd, hdc) ;
5.3 点和线的绘制
5.3.1 设定像素
SetPixel函数在指定的x和y坐标以特定的颜色设定图素:
SetPixel (hdc, x, y, crColor) ;
第一个参数是设备内容的句柄。第二个和第三个参数指明了坐标位置。通常要获得窗口显示区域的设备内容,并且x和y相对于该显示区域的左上角。最后一个参数是COLORREF型态指定了颜色。如果在函数中指定的颜色视讯显示器不支持,则函数将图素设定为最接近的纯色并从函数传回该值。
GetPixel函数传回指定坐标处的图素颜色:
crColor = GetPixel (hdc, x, y) ;
5.3.2 直线
画一条直线,必须呼叫两个函数。第一个函数指定了线的开始点,第二个函数指定了线的终点:
MoveToEx (hdc, xBeg, yBeg, NULL) ;
LineTo (hdc, xEnd, yEnd) ;
绘制直线
#include
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);//消息函数声明
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)//主函数
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");//窗口类名称
HWND hwnd;//句柄
MSG msg;//结构体
WNDCLASS wndclass;//窗口类
//窗口类属性
wndclass.style = CS_HREDRAW | CS_VREDRAW;//样式
wndclass.lpfnWndProc = WndProc;//窗口处理函数
wndclass.cbClsExtra = 0;//窗口实例扩展
wndclass.cbWndExtra = 0;//窗口类扩展
wndclass.hInstance = hInstance;//窗口实例句柄
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);//加载图标
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);//鼠标,移入内容区域变成箭头
wndclass.hbrBackground = (HBRUSH)GetStockObject(DKGRAY_BRUSH);//主窗口背景色
wndclass.lpszMenuName = NULL;//窗口菜单
wndclass.lpszClassName = szAppName;//窗口类名
if (!RegisterClass(&wndclass)) {//注册窗口类,如果注册失败弹出窗口
MessageBox(NULL, TEXT("窗口创建失败!程序需要Windows NT!(传递窗口消息为UNICODE)"), szAppName, MB_ICONERROR);//消息窗口
return 0;
}
hwnd = CreateWindow(szAppName, //Windows类名
TEXT("窗口绘制成功!"), //窗口标题
WS_OVERLAPPEDWINDOW, //窗口风格
CW_USEDEFAULT, //初始化窗口位置的X坐标
CW_USEDEFAULT, //初始化窗口位置的Y坐标
500, //初始化窗口宽度大小
500, //初始化窗口长度大小
NULL, //父类窗口句柄
NULL, //窗口菜单句柄
hInstance, //程序实例句柄
NULL); //创建参数
ShowWindow(hwnd, iCmdShow);//显示窗口
UpdateWindow(hwnd);//更新窗口
while (GetMessage(&msg, NULL, 0, 0)) {//从消息队列中获取消息
TranslateMessage(&msg);//将虚拟键消息转换为字符消息
DispatchMessage(&msg);//分发到回调函数
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
HDC hdc;//设备环境句柄
int x, y;
/*
typedef struct tagRECT
{
LONG left;
LONG top;
LONG right;
LONG bottom;
} RECT
其中left,top赋为0,因此right和bottom表示客户区的宽度和高度(像素)
*/
RECT rect;//矩形结构
switch (message) {//处理得到的消息
case WM_PAINT://处理窗口绘制
hdc = GetDC(hwnd);
GetClientRect(hwnd, &rect);//获取当前位置
for (x = 0; x 50) {//竖线
MoveToEx(hdc, x, 0, NULL);//设置起点
LineTo(hdc, x, rect.bottom);//设置终点
}
for (y = 0; y 50) {//横线
MoveToEx(hdc, 0, y, NULL);
LineTo(hdc, rect.right, y);
}
ReleaseDC(hwnd, hdc);
return 0;
case WM_DESTROY://处理窗口关闭时的消息
PostQuitMessage(0);//将退出消息插入消息队列,程序从消息循环退出,return msg.wParam
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);//执行默认消息处理
}
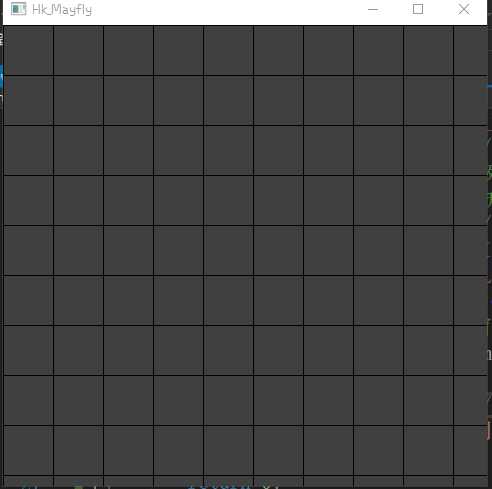
绘制矩形


#include
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);//消息函数声明
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)//主函数
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");//窗口类名称
HWND hwnd;//句柄
MSG msg;//结构体
WNDCLASS wndclass;//窗口类
//窗口类属性
wndclass.style = CS_HREDRAW | CS_VREDRAW;//样式
wndclass.lpfnWndProc = WndProc;//窗口处理函数
wndclass.cbClsExtra = 0;//窗口实例扩展
wndclass.cbWndExtra = 0;//窗口类扩展
wndclass.hInstance = hInstance;//窗口实例句柄
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);//加载图标
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);//鼠标,移入内容区域变成箭头
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITENESS);//主窗口背景色
wndclass.lpszMenuName = NULL;//窗口菜单
wndclass.lpszClassName = szAppName;//窗口类名
if (!RegisterClass(&wndclass)) {//注册窗口类,如果注册失败弹出窗口
MessageBox(NULL, TEXT("窗口创建失败!程序需要Windows NT!(传递窗口消息为UNICODE)"), szAppName, MB_ICONERROR);//消息窗口
return 0;
}
hwnd = CreateWindow(szAppName, //Windows类名
TEXT("Hk_Mayfly"), //窗口标题
WS_OVERLAPPEDWINDOW, //窗口风格
CW_USEDEFAULT, //初始化窗口位置的X坐标
CW_USEDEFAULT, //初始化窗口位置的Y坐标
500, //初始化窗口宽度大小
500, //初始化窗口长度大小
NULL, //父类窗口句柄
NULL, //窗口菜单句柄
hInstance, //程序实例句柄
NULL); //创建参数
ShowWindow(hwnd, iCmdShow);//显示窗口
UpdateWindow(hwnd);//更新窗口
while (GetMessage(&msg, NULL, 0, 0)) {//从消息队列中获取消息
TranslateMessage(&msg);//将虚拟键消息转换为字符消息
DispatchMessage(&msg);//分发到回调函数
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
HDC hdc;//设备环境句柄
POINT apt[5] = { 100, 100, 200, 100, 200, 200, 100, 200, 100, 100 };//矩形坐标
int i;
/*
typedef struct tagRECT
{
LONG left;
LONG top;
LONG right;
LONG bottom;
} RECT
其中left,top赋为0,因此right和bottom表示客户区的宽度和高度(像素)
*/
RECT rect;//矩形结构
switch (message) {//处理得到的消息
case WM_PAINT://处理窗口绘制
hdc = GetDC(hwnd);
GetClientRect(hwnd, &rect);//获取当前位置
MoveToEx(hdc, apt[0].x, apt[0].y, NULL);
for (i = 1; i 5; ++i) {
LineTo(hdc, apt[i].x, apt[i].y);
}
ReleaseDC(hwnd, hdc);
return 0;
case WM_DESTROY://处理窗口关闭时的消息
PostQuitMessage(0);//将退出消息插入消息队列,程序从消息循环退出,return msg.wParam
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);//执行默认消息处理
}
View Code
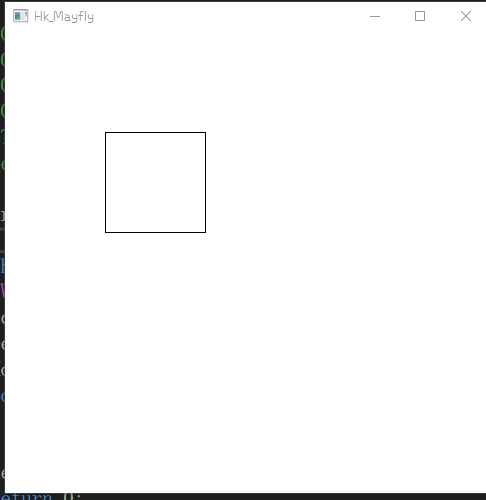
使用Polyline替换,也可以绘制矩形。
或者
MoveToEx(hdc, apt[0].x, apt[0].y, NULL);
PolylineTo(hdc, apt, 5);
绘制正弦曲线


#include
#include #define NUM 1000
#define TWOPI (2*3.14159)
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);//消息函数声明
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)//主函数
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");//窗口类名称
HWND hwnd;//句柄
MSG msg;//结构体
WNDCLASS wndclass;//窗口类
//窗口类属性
wndclass.style = CS_HREDRAW | CS_VREDRAW;//样式
wndclass.lpfnWndProc = WndProc;//窗口处理函数
wndclass.cbClsExtra = 0;//窗口实例扩展
wndclass.cbWndExtra = 0;//窗口类扩展
wndclass.hInstance = hInstance;//窗口实例句柄
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);//加载图标
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);//鼠标,移入内容区域变成箭头
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITENESS);//主窗口背景色
wndclass.lpszMenuName = NULL;//窗口菜单
wndclass.lpszClassName = szAppName;//窗口类名
if (!RegisterClass(&wndclass)) {//注册窗口类,如果注册失败弹出窗口
MessageBox(NULL, TEXT("窗口创建失败!程序需要Windows NT!(传递窗口消息为UNICODE)"), szAppName, MB_ICONERROR);//消息窗口
return 0;
}
hwnd = CreateWindow(szAppName, //Windows类名
TEXT("Hk_Mayfly"), //窗口标题
WS_OVERLAPPEDWINDOW, //窗口风格
CW_USEDEFAULT, //初始化窗口位置的X坐标
CW_USEDEFAULT, //初始化窗口位置的Y坐标
1000, //初始化窗口宽度大小
500, //初始化窗口高度大小
NULL, //父类窗口句柄
NULL, //窗口菜单句柄
hInstance, //程序实例句柄
NULL); //创建参数
ShowWindow(hwnd, iCmdShow);//显示窗口
UpdateWindow(hwnd);//更新窗口
while (GetMessage(&msg, NULL, 0, 0)) {//从消息队列中获取消息
TranslateMessage(&msg);//将虚拟键消息转换为字符消息
DispatchMessage(&msg);//分发到回调函数
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
HDC hdc;//设备环境句柄
PAINTSTRUCT ps;
static int cxClient, cyClient;
int i;
POINT apt[NUM];
switch (message) {//处理得到的消息
case WM_SIZE:
cxClient = LOWORD(lParam);//客户区宽度
cyClient = HIWORD(lParam);//客户区的高度
return 0;
case WM_PAINT://处理窗口绘制
hdc = BeginPaint(hwnd, &ps);
MoveToEx(hdc, 0, cyClient / 2, NULL);//中间横线
LineTo(hdc, cxClient, cyClient / 2);
for (i = 0; i ) {
apt[i].x = i * cxClient / NUM;
apt[i].y = (int)(cyClient / 2 * (1 - sin(TWOPI * i / NUM)));
//apt[i].x = i*15;
//apt[i].y = (int)cyClient*sin(0.1*i);
}
Polyline(hdc, apt, NUM);
EndPaint(hwnd, &ps);
return 0;
case WM_DESTROY://处理窗口关闭时的消息
PostQuitMessage(0);//将退出消息插入消息队列,程序从消息循环退出,return msg.wParam
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);//执行默认消息处理
}
View Code
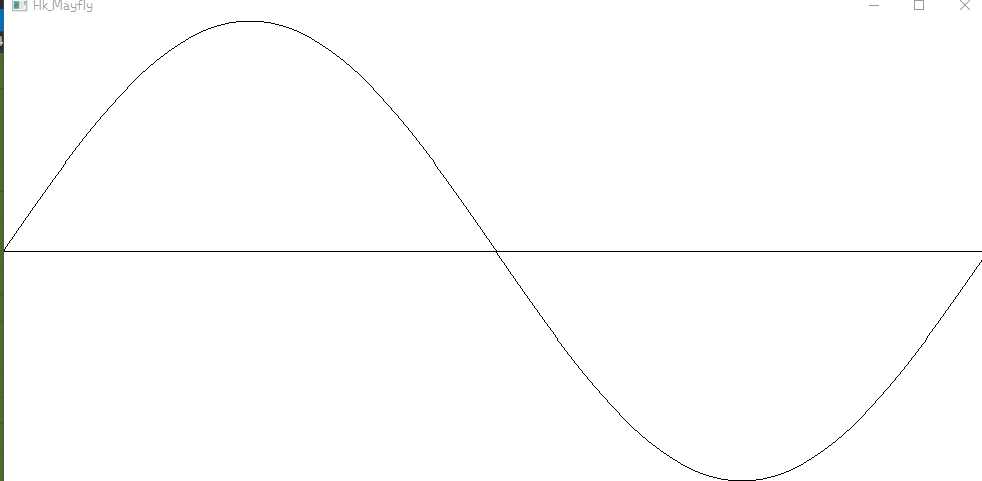
5.3.3 边框绘制函数


#include
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if (!RegisterClass(&wndclass)) {
MessageBox(NULL, TEXT("窗口创建失败!需要Windows NT!(传递消息为UNICODE)"), szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(
szAppName,
TEXT("Hk_Mayfly"),
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,
CW_USEDEFAULT,
1000,//宽度
500,//高度
NULL,
NULL,
hInstance,
NULL
);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
HDC hdc;
PAINTSTRUCT ps;
switch (message) {
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
//Rectangle(hdc, 100, 200, 500, 400);//矩形
//Ellipse(hdc, 100, 200, 500, 400);//椭圆
//RoundRect(hdc, 100, 200, 500, 400, 100, 100);//圆角矩阵
//Arc(hdc, 100, 200, 500, 400, 500, 350, 100, 350);//弧
//Chord(hdc, 100, 200, 500, 400, 500, 350, 100, 350);//弦
//Pie(hdc, 100, 200, 500, 400, 500, 350, 100, 350);//饼
EndPaint(hwnd, &ps);
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
View Code
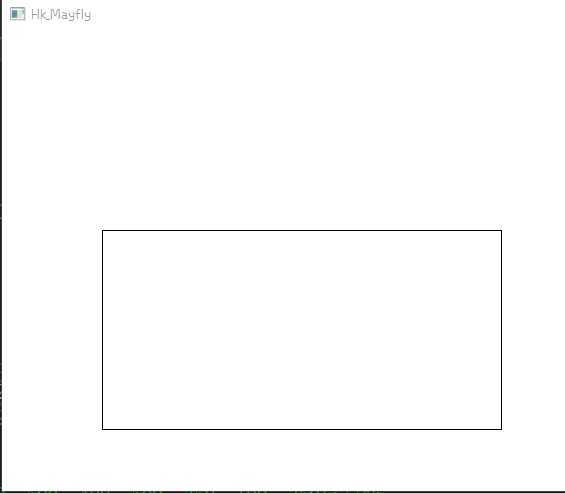
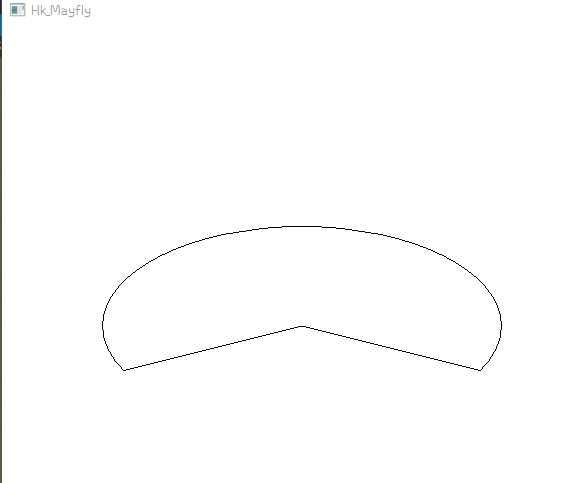
自定义程序
#include
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if (!RegisterClass(&wndclass)) {
MessageBox(NULL, TEXT("窗口创建失败!需要Windows NT!(传递消息为UNICODE)"), szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(
szAppName,
TEXT("Hk_Mayfly"),
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,
CW_USEDEFAULT,
800,//宽度
800,//高度
NULL,
NULL,
hInstance,
NULL
);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
static int cxClient, cyClient;
HDC hdc;
PAINTSTRUCT ps;
switch (message) {
case WM_SIZE:
cxClient = LOWORD(lParam);
cyClient = HIWORD(lParam);
return 0;
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
Rectangle(hdc, 200, 200, 600, 600);
MoveToEx(hdc, 0, 0, NULL);
LineTo(hdc, 200, 200);
MoveToEx(hdc, cxClient, 0, NULL);
LineTo(hdc, 600, 200);
MoveToEx(hdc, 0, cyClient, NULL);
LineTo(hdc, 200, 600);
MoveToEx(hdc, cxClient, cyClient, NULL);
LineTo(hdc, 600, 600);
Ellipse(hdc, 200, 200, 600, 600);
Arc(hdc, 300, 200, 500, 400, 400, 200, 400, 400);//弧,逆时针绘制
Arc(hdc, 300, 400, 500, 600, 400, 600, 400, 400);
Ellipse(hdc, 388, 288, 412, 312);//小圆圈
Ellipse(hdc, 388, 488, 412, 512);
EndPaint(hwnd, &ps);
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
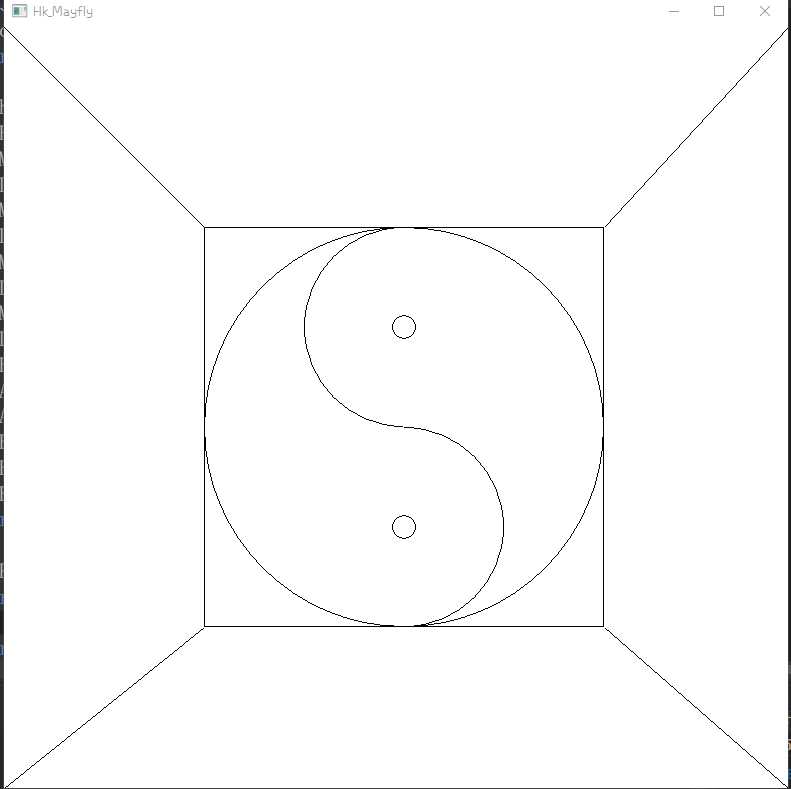
5.3.4 贝塞尔样条曲线
一条二维的贝塞尔曲线由四个点定义-两个端点和两个控制点。曲线的端点在两个端点上,控制点就好像「磁石」一样把曲线从两个端点间的直线处拉走。


#include
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if (!RegisterClass(&wndclass)) {
MessageBox(NULL, TEXT("窗口创建失败!需要Windows NT!(传递消息为UNICODE)"), szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(
szAppName,
TEXT("Hk_Mayfly"),
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,
CW_USEDEFAULT,
CW_USEDEFAULT,//宽度
CW_USEDEFAULT,//高度
NULL,
NULL,
hInstance,
NULL
);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
void DrawBezier(HDC hdc, POINT apt[]) {
PolyBezier(hdc, apt, 4);
MoveToEx(hdc, apt[0].x, apt[0].y, NULL);
LineTo(hdc, apt[1].x, apt[1].y);
MoveToEx(hdc, apt[2].x, apt[2].y, NULL);
LineTo(hdc, apt[3].x, apt[3].y);
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
static POINT apt[4];
int cxClient, cyClient;
HDC hdc;
PAINTSTRUCT ps;
switch (message) {
case WM_SIZE:
cxClient = LOWORD(lParam);
cyClient = HIWORD(lParam);
apt[0].x = cxClient / 4;
apt[0].y = cyClient / 2;
apt[1].x = cxClient / 2;
apt[1].y = cyClient / 4;
apt[2].x = cxClient / 2;
apt[2].y = 3 * cyClient / 4;
apt[3].x = 3 * cxClient / 4;
apt[3].y = cyClient / 2;
return 0;
case WM_LBUTTONDOWN:
case WM_RBUTTONDOWN:
case WM_MOUSEMOVE:
if (wParam & MK_LBUTTON || wParam & MK_RBUTTON) {
hdc = GetDC(hwnd);
SelectObject(hdc, GetStockObject(WHITE_PEN));
DrawBezier(hdc, apt);
if (wParam & MK_LBUTTON) {
apt[1].x = LOWORD(lParam);
apt[1].y = HIWORD(lParam);
}
if (wParam & MK_RBUTTON) {
apt[2].x = LOWORD(lParam);
apt[2].y = HIWORD(lParam);
}
SelectObject(hdc, GetStockObject(BLACK_PEN));
DrawBezier(hdc, apt);
ReleaseDC(hwnd, hdc);
}
return 0;
case WM_PAINT:
InvalidateRect(hwnd, NULL, TRUE);
hdc = BeginPaint(hwnd, &ps);
DrawBezier(hdc, apt);
EndPaint(hwnd, &ps);
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
View Code
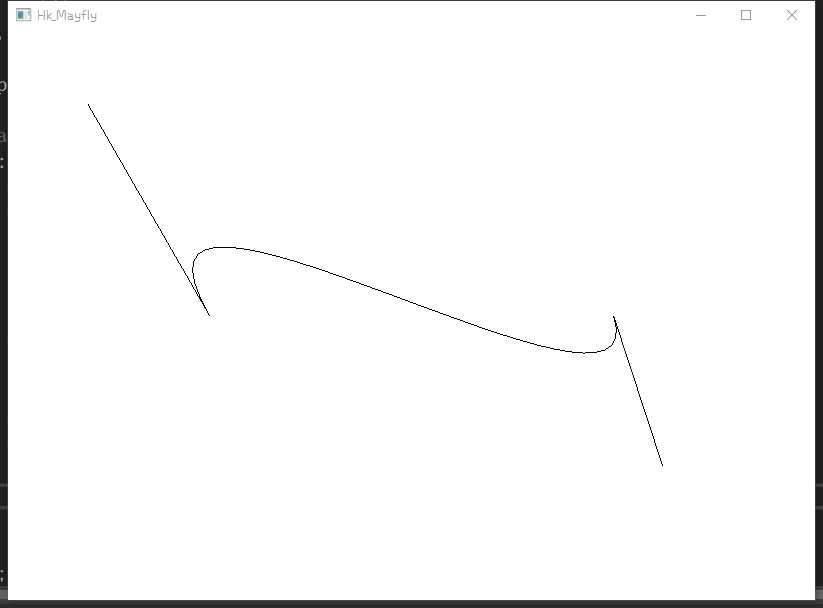
5.3.6 创建,选择和删除画笔
#include
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if (!RegisterClass(&wndclass)) {
MessageBox(NULL, TEXT("窗口创建失败!需要Windows NT!(传递消息为UNICODE)"), szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(
szAppName,
TEXT("Hk_Mayfly"),
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,
CW_USEDEFAULT,
800,//宽度
800,//高度
NULL,
NULL,
hInstance,
NULL
);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
static int cxClient, cyClient;
HDC hdc;
PAINTSTRUCT ps;
static HPEN hPen1, hPen2;
switch (message) {
case WM_CREATE:
hPen1 = CreatePen(PS_DOT, 0, 0);
hPen2 = CreatePen(PS_DASH, 1, RGB(255, 0, 0));
case WM_SIZE:
cxClient = LOWORD(lParam);
cyClient = HIWORD(lParam);
return 0;
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
SelectObject(hdc, hPen1);
Ellipse(hdc, 200, 200, 600, 600);
Ellipse(hdc, 388, 288, 412, 312);//小圆圈
SelectObject(hdc, hPen2);
Arc(hdc, 300, 200, 500, 400, 400, 200, 400, 400);//弧,逆时针绘制
SelectObject(hdc, CreatePen(PS_DASH, 1, RGB(50, 50, 50)));
Arc(hdc, 300, 400, 500, 600, 400, 600, 400, 400);
Ellipse(hdc, 388, 488, 412, 512);
DeleteObject(SelectObject(hdc, CreatePen(PS_DASH, 1, RGB(50, 50, 50))));
EndPaint(hwnd, &ps);
return 0;
case WM_DESTROY:
DeleteObject(hPen1);
DeleteObject(hPen2);
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
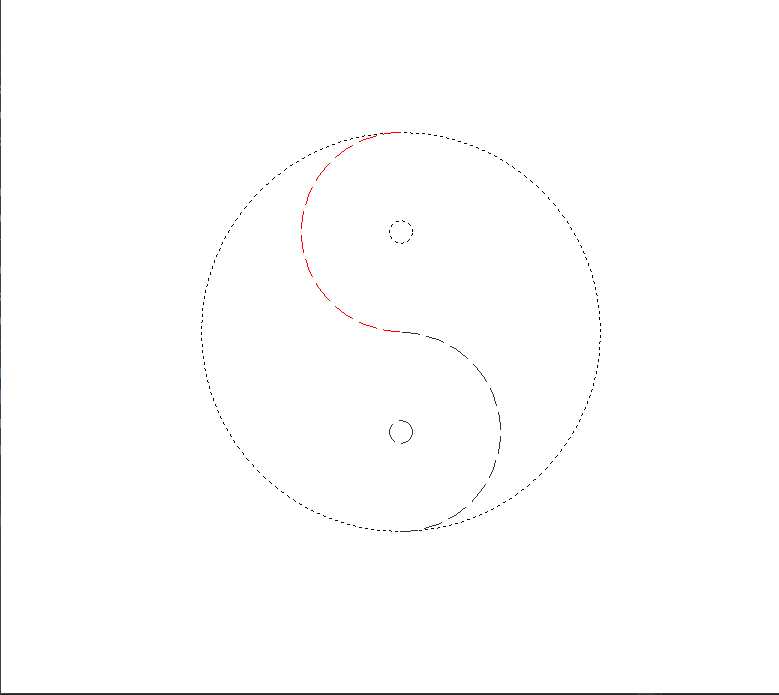
5.4 绘制填充区域


#include
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if (!RegisterClass(&wndclass)) {
MessageBox(NULL, TEXT("窗口创建失败!需要Windows NT!(传递消息为UNICODE)"), szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(
szAppName,
TEXT("Hk_Mayfly"),
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,
CW_USEDEFAULT,
800,//宽度
800,//高度
NULL,
NULL,
hInstance,
NULL
);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
static int cxClient, cyClient;
HDC hdc;
PAINTSTRUCT ps;
static HBRUSH hBrush;
switch (message) {
case WM_CREATE:
hBrush = GetStockObject(LTGRAY_BRUSH);//获取句柄
case WM_SIZE:
cxClient = LOWORD(lParam);
cyClient = HIWORD(lParam);
return 0;
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
SelectObject(hdc, hBrush);//选入设备环境
Ellipse(hdc, 200, 200, 600, 600);
Ellipse(hdc, 388, 288, 412, 312);//小圆圈
Arc(hdc, 300, 200, 500, 400, 400, 200, 400, 400);//弧,逆时针绘制
Arc(hdc, 300, 400, 500, 600, 400, 600, 400, 400);
Ellipse(hdc, 388, 488, 412, 512);
DeleteObject(SelectObject(hdc, CreatePen(PS_DASH, 1, RGB(50, 50, 50))));
EndPaint(hwnd, &ps);
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
View Code
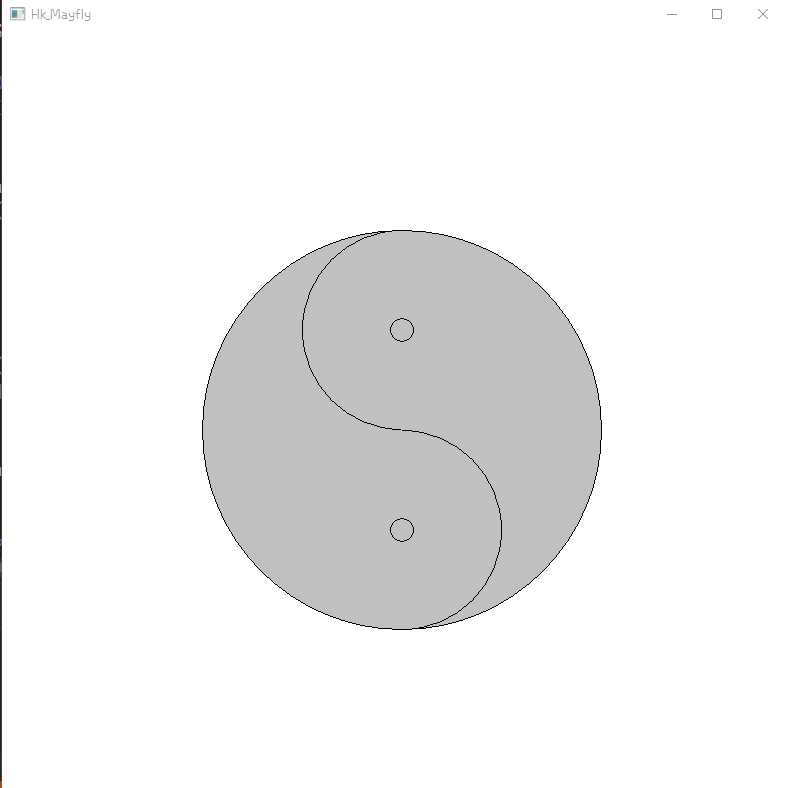
5.4.1 Polygon函数和多边形填充模式
Polygon按照点的顺序连接多边图形,如果数组中最后一个点与第一个点不同,则Windows会再添加一条线连接最后一个点与第一个点。
#include
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if (!RegisterClass(&wndclass)) {
MessageBox(NULL, TEXT("窗口创建失败!需要Windows NT!(传递消息为UNICODE)"), szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(
szAppName,
TEXT("Hk_Mayfly"),
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,
CW_USEDEFAULT,
CW_USEDEFAULT,//宽度
CW_USEDEFAULT,//高度
NULL,
NULL,
hInstance,
NULL
);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam) {
static POINT apt[6] = { 100, 200, 100, 100, 200, 50, 300, 100, 300, 200, 200, 250};
static int cxClient, cyClient;
HDC hdc;
PAINTSTRUCT ps;
switch (message) {
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
SelectObject(hdc, GetStockObject(GRAY_BRUSH));//获取灰色画刷并设置到环境设备中
Polygon(hdc, apt, 6);
EndPaint(hwnd, &ps);
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
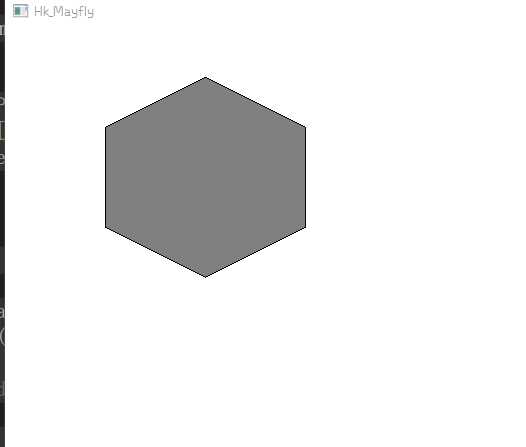
PolyPolygon(hdc, apt, aiCounts, iPolyCount);
#include
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("WNDCLASS NAME");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_B