标签:时间 get rip mod validate winform LTP img edr
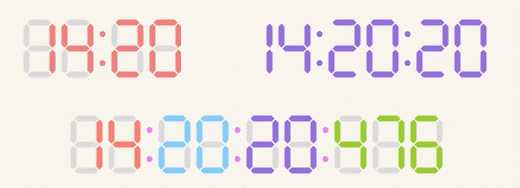
///
/// 数字时间控件
///
[ToolboxItem(true)]
[DefaultProperty("Value")]
[DefaultEvent("ValueChanged")]
[Description("数字时间控件")]
public partial class TimeExt : Control
{
public delegate void EventHandler(object sender, TimeEventArgs e);
private event EventHandler valueChanged;
///
/// 时间值更改事件
///
[Description("时间值更改事件")]
public event EventHandler ValueChanged
{
add { this.valueChanged += value; }
remove { this.valueChanged -= value; }
}
#region
private int lineWidth = 6;
///
/// 线宽度(必须偶数)
///
[DefaultValue(6)]
[Description("线宽度(必须偶数)")]
public int LineWidth
{
get { return this.lineWidth; }
set
{
if (this.lineWidth == value || value % 2 != 0)
return;
this.lineWidth = value;
this.InitializeRectangle();
this.Invalidate();
}
}
private Color lineHighlightColor = Color.FromArgb(147, 112, 219);
///
/// 线高亮颜色
///
[DefaultValue(typeof(Color), "147, 112, 219")]
[Description("线高亮颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color LineHighlightColor
{
get { return this.lineHighlightColor; }
set
{
if (this.lineHighlightColor == value)
return;
this.lineHighlightColor = value;
if (this.HourLineHighlightColor == Color.Empty)
this.highlight_hour_pen.Color = value;
if (this.MinuteLineHighlightColor == Color.Empty)
this.highlight_minute_pen.Color = value;
if (this.SecondLineHighlightColor == Color.Empty)
this.highlight_second_pen.Color = value;
if (this.MillisecondLineHighlightColor == Color.Empty)
this.highlight_millisecond_pen.Color = value;
this.split_sb.Color = value;
this.Invalidate();
}
}
private bool shadowShow = true;
///
/// 是否显示线阴影
///
[DefaultValue(true)]
[Description("是否显示线阴影")]
public bool ShadowShow
{
get { return this.shadowShow; }
set
{
if (this.shadowShow == value)
return;
this.shadowShow = value;
this.Invalidate();
}
}
private Color lineShadowColor = Color.FromArgb(220, 220, 220);
///
/// 线阴影颜色
///
[DefaultValue(typeof(Color), "220, 220, 220")]
[Description("线阴影颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color LineShadowColor
{
get { return this.lineShadowColor; }
set
{
if (this.lineShadowColor == value)
return;
this.lineShadowColor = value;
this.Invalidate();
}
}
private Color hourLineHighlightColor = Color.Empty;
///
/// 小时高亮颜色
///
[DefaultValue(typeof(Color), "Empty")]
[Description("小时高亮颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color HourLineHighlightColor
{
get { return this.hourLineHighlightColor; }
set
{
if (this.hourLineHighlightColor == value)
return;
this.hourLineHighlightColor = value;
if (value == Color.Empty)
this.highlight_hour_pen.Color = this.LineHighlightColor;
else
this.highlight_hour_pen.Color = value;
this.Invalidate();
}
}
private Color minuteLineHighlightColor = Color.Empty;
///
/// 分钟高亮颜色
///
[DefaultValue(typeof(Color), "Empty")]
[Description("分钟高亮颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color MinuteLineHighlightColor
{
get { return this.minuteLineHighlightColor; }
set
{
if (this.minuteLineHighlightColor == value)
return;
this.minuteLineHighlightColor = value;
if (value == Color.Empty)
this.highlight_minute_pen.Color = this.LineHighlightColor;
else
this.highlight_minute_pen.Color = value;
this.Invalidate();
}
}
private Color secondLineHighlightColor = Color.Empty;
///
/// 秒高亮颜色
///
[DefaultValue(typeof(Color), "Empty")]
[Description("秒高亮颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color SecondLineHighlightColor
{
get { return this.secondLineHighlightColor; }
set
{
if (this.secondLineHighlightColor == value)
return;
this.secondLineHighlightColor = value;
if (value == Color.Empty)
this.highlight_second_pen.Color = this.LineHighlightColor;
else
this.highlight_second_pen.Color = value;
this.Invalidate();
}
}
private Color millisecondLineHighlightColor = Color.Empty;
///
/// 毫秒高亮颜色
///
[DefaultValue(typeof(Color), "Empty")]
[Description("毫秒高亮颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color MillisecondLineHighlightColor
{
get { return this.millisecondLineHighlightColor; }
set
{
if (this.millisecondLineHighlightColor == value)
return;
this.millisecondLineHighlightColor = value;
if (value == Color.Empty)
this.highlight_millisecond_pen.Color = this.LineHighlightColor;
else
this.highlight_millisecond_pen.Color = value;
this.Invalidate();
}
}
private DateTime value;
///
/// 时间
///
[Description("时间")]
public DateTime Value
{
get { return this.value; }
set
{
if (this.value == value)
return;
this.value = value;
if (this.valueChanged != null)
{
this.valueChanged(this, new TimeEventArgs() { Value = value });
}
this.Invalidate();
}
}
private TimeType timeTypeFormat = TimeType.HourMinuteSecond;
///
/// 时间显示格式
///
[DefaultValue(TimeType.HourMinuteSecond)]
[Description("时间显示格式")]
public TimeType TimeTypeFormat
{
get { return this.timeTypeFormat; }
set
{
if (this.timeTypeFormat == value)
return;
this.timeTypeFormat = value;
this.InitializeRectangle();
this.Invalidate();
}
}
protected override Size DefaultSize
{
get
{
return new Size(280, 67);
}
}
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public override Color BackColor
{
get
{
return base.BackColor;
}
set
{
base.BackColor = value;
this.Invalidate();
}
}
#endregion
#region
///
/// 笔帽的一半
///
private float cap_c = 0f;
///
/// 数字笔画横向宽度
///
private float line_w = 0f;
///
/// 数字笔画纵向高度
///
private float line_h = 0f;
private RectangleF hour1_rect;
private RectangleF hour2_rect;
private RectangleF split1_rect;
private RectangleF minute1_rect;
private RectangleF minute2_rect;
private RectangleF split2_rect;
private RectangleF second1_rect;
private RectangleF second2_rect;
private RectangleF split3_rect;
private RectangleF millisecond1_rect;
private RectangleF millisecond2_rect;
private RectangleF millisecond3_rect;
private Pen highlight_hour_pen;
private Pen highlight_minute_pen;
private Pen highlight_second_pen;
private Pen highlight_millisecond_pen;
private Pen shadow_pen;
private SolidBrush split_sb;
#endregion
public TimeExt()
{
SetStyle(ControlStyles.UserPaint, true);
SetStyle(ControlStyles.AllPaintingInWmPaint, true);
SetStyle(ControlStyles.OptimizedDoubleBuffer, true);
SetStyle(ControlStyles.ResizeRedraw, true);
SetStyle(ControlStyles.SupportsTransparentBackColor, true);
InitializeComponent();
this.highlight_hour_pen = new Pen(this.HourLineHighlightColor == Color.Empty ? this.LineHighlightColor : this.HourLineHighlightColor, this.LineWidth);
this.highlight_hour_pen.StartCap = LineCap.Triangle;
this.highlight_hour_pen.EndCap = LineCap.Triangle;
this.highlight_minute_pen = new Pen(this.MinuteLineHighlightColor == Color.Empty ? this.LineHighlightColor : this.MinuteLineHighlightColor, this.LineWidth);
this.highlight_minute_pen.StartCap = LineCap.Triangle;
this.highlight_minute_pen.EndCap = LineCap.Triangle;
this.highlight_second_pen = new Pen(this.SecondLineHighlightColor == Color.Empty ? this.LineHighlightColor : this.SecondLineHighlightColor, this.LineWidth);
this.highlight_second_pen.StartCap = LineCap.Triangle;
this.highlight_second_pen.EndCap = LineCap.Triangle;
this.highlight_millisecond_pen = new Pen(this.MillisecondLineHighlightColor == Color.Empty ? this.LineHighlightColor : this.MillisecondLineHighlightColor, this.LineWidth);
this.highlight_millisecond_pen.StartCap = LineCap.Triangle;
this.highlight_millisecond_pen.EndCap = LineCap.Triangle;
this.shadow_pen = new Pen(this.lineShadowColor, this.LineWidth);
this.shadow_pen.StartCap = LineCap.Triangle;
this.shadow_pen.EndCap = LineCap.Triangle;
this.split_sb = new SolidBrush(this.lineHighlightColor);
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
if (this.Value == null)
return;
Graphics g = e.Graphics;
g.SmoothingMode = SmoothingMode.AntiAlias;
RectangleF bounds_rect = g.ClipBounds;
if (this.TimeTypeFormat == TimeType.Hour)
{
this.draw_num(g, this.highlight_hour_pen, this.hour1_rect, this.shadow_pen, int.Parse(this.Value.ToString("HH").Substring(0, 1)));
this.draw_num(g, this.highlight_hour_pen, this.hour2_rect, this.shadow_pen, int.Parse(this.Value.ToString("HH").Substring(1, 1)));
}
else if (this.TimeTypeFormat == TimeType.HourMinute)
{
this.draw_num(g, this.highlight_hour_pen, this.hour1_rect, this.shadow_pen, int.Parse(this.Value.ToString("HH").Substring(0, 1)));
this.draw_num(g, this.highlight_hour_pen, this.hour2_rect, this.shadow_pen, int.Parse(this.Value.ToString("HH").Substring(1, 1)));
this.draw_split(g, split_sb, this.split1_rect);
this.draw_num(g, this.highlight_minute_pen, this.minute1_rect, this.shadow_pen, int.Parse(this.Value.ToString("mm").Substring(0, 1)));
this.draw_num(g, this.highlight_minute_pen, this.minute2_rect, this.shadow_pen, int.Parse(this.Value.ToString("mm").Substring(1, 1)));
}
else if (this.TimeTypeFormat == TimeType.HourMinuteSecond)
{
this.draw_num(g, this.highlight_hour_pen, this.hour1_rect, this.shadow_pen, int.Parse(this.Value.ToString("HH").Substring(0, 1)));
this.draw_num(g, this.highlight_hour_pen, this.hour2_rect, this.shadow_pen, int.Parse(this.Value.ToString("HH").Substring(1, 1)));
this.draw_split(g, split_sb, this.split1_rect);
this.draw_num(g, this.highlight_minute_pen, this.minute1_rect, this.shadow_pen, int.Parse(this.Value.ToString("mm").Substring(0, 1)));
this.draw_num(g, this.highlight_minute_pen, this.minute2_rect, this.shadow_pen, int.Parse(this.Value.ToString("mm").Substring(1, 1)));
this.draw_split(g, split_sb, this.split2_rect);
this.draw_num(g, this.highlight_second_pen, this.second1_rect, this.shadow_pen, int.Parse(this.Value.ToString("ss").Substring(0, 1)));
this.draw_num(g, this.highlight_second_pen, this.second2_rect, this.shadow_pen, int.Parse(this.Value.ToString("ss").Substring(1, 1)));
}
else if (this.TimeTypeFormat == TimeType.HourMinuteSecondMillisecond)
{
this.draw_num(g, this.highlight_hour_pen, this.hour1_rect, this.shadow_pen, int.Parse(this.Value.ToString("HH").Substring(0, 1)));
this.draw_num(g, this.highlight_hour_pen, this.hour2_rect, this.shadow_pen, int.Parse(this.Value.ToString("HH").Substring(1, 1)));
this.draw_split(g, split_sb, this.split1_rect);
this.draw_num(g, this.highlight_minute_pen, this.minute1_rect, this.shadow_pen, int.Parse(this.Value.ToString("mm").Substring(0, 1)));
this.draw_num(g, this.highlight_minute_pen, this.minute2_rect, this.shadow_pen, int.Parse(this.Value.ToString("mm").Substring(1, 1)));
this.draw_split(g, split_sb, this.split2_rect);
this.draw_num(g, this.highlight_second_pen, this.second1_rect, this.shadow_pen, int.Parse(this.Value.ToString("ss").Substring(0, 1)));
this.draw_num(g, this.highlight_second_pen, this.second2_rect, this.shadow_pen, int.Parse(this.Value.ToString("ss").Substring(1, 1)));
this.draw_split(g, split_sb, this.split3_rect);
this.draw_num(g, this.highlight_millisecond_pen, this.millisecond1_rect, this.shadow_pen, int.Parse(this.Value.ToString("fff").Substring(0, 1)));
this.draw_num(g, this.highlight_millisecond_pen, this.millisecond2_rect, this.shadow_pen, int.Parse(this.Value.ToString("fff").Substring(1, 1)));
this.draw_num(g, this.highlight_millisecond_pen, this.millisecond3_rect, this.shadow_pen, int.Parse(this.Value.ToString("fff").Substring(2, 1)));
}
}
protected override void OnResize(EventArgs e)
{
base.OnResize(e);
this.InitializeRectangle();
}
///
/// 初始化数字rect
///
private void InitializeRectangle()
{
this.cap_c = (float)this.LineWidth / 2;
this.line_w = (float)this.LineWidth * 4;
this.line_h = this.line_w;
float rectf_w = this.line_w + this.LineWidth + 2;
float rectf_h = this.line_h * 2 + this.LineWidth + 4;
float split_w = this.LineWidth * 3;
float start_x = 0f;
if (this.TimeTypeFormat == TimeType.Hour)
{
start_x = (this.ClientRectangle.Width - (rectf_w * 2 + this.LineWidth)) / 2;
}
else if (this.TimeTypeFormat == TimeType.HourMinute)
{
start_x = (this.ClientRectangle.Width - (rectf_w * 4 + this.LineWidth * 2 + split_w)) / 2;
}
else if (this.TimeTypeFormat == TimeType.HourMinuteSecond)
{
start_x = (this.ClientRectangle.Width - (rectf_w * 6 + this.LineWidth * 3 + split_w * 2)) / 2;
}
else if (this.TimeTypeFormat == TimeType.HourMinuteSecondMillisecond)
{
start_x = (this.ClientRectangle.Width - (rectf_w * 9 + this.LineWidth * 5 + split_w * 3)) / 2;
}
float start_y = (this.ClientRectangle.Height - rectf_h) / 2;
this.hour1_rect = new RectangleF(start_x, start_y, rectf_w, rectf_h);
this.hour2_rect = new RectangleF(this.hour1_rect.Right + this.LineWidth, start_y, rectf_w, rectf_h);
this.split1_rect = new RectangleF(this.hour2_rect.Right, start_y, split_w, rectf_h);
this.minute1_rect = new RectangleF(this.split1_rect.Right, start_y, rectf_w, rectf_h);
this.minute2_rect = new RectangleF(this.minute1_rect.Right + this.LineWidth, start_y, rectf_w, rectf_h);
this.split2_rect = new RectangleF(this.minute2_rect.Right, start_y, split_w, rectf_h);
this.second1_rect = new RectangleF(this.split2_rect.Right, start_y, rectf_w, rectf_h);
this.second2_rect = new RectangleF(this.second1_rect.Right + this.LineWidth, start_y, rectf_w, rectf_h);
this.split3_rect = new RectangleF(this.second2_rect.Right, start_y, split_w, rectf_h);
this.millisecond1_rect = new RectangleF(this.split3_rect.Right, start_y, rectf_w, rectf_h);
this.millisecond2_rect = new RectangleF(this.millisecond1_rect.Right + this.LineWidth, start_y, rectf_w, rectf_h);
this.millisecond3_rect = new RectangleF(this.millisecond2_rect.Right + this.LineWidth, start_y, rectf_w, rectf_h);
}
#region 绘制数字笔画
//
// --- 1
// | | 11 12
// --- 2
// | | 21 22
// --- 3
//
private void draw_vertical_11(Graphics g, Pen pen, RectangleF bounds_rect)
{
float x1 = bounds_rect.X + this.cap_c;
float y1 = bounds_rect.Y + this.LineWidth + 1;
float x2 = bounds_rect.X + this.cap_c;
float y2 = bounds_rect.Y + this.line_h + 1;
g.DrawLine(pen, x1, y1, x2, y2);
}
private void draw_vertical_21(Graphics g, Pen pen, RectangleF bounds_rect)
{
float x1 = bounds_rect.X + this.cap_c;
float y1 = bounds_rect.Y + this.LineWidth + this.line_h + 3;
float x2 = bounds_rect.X + this.cap_c;
float y2 = bounds_rect.Y + this.line_h + this.line_h + 3;
g.DrawLine(pen, x1, y1, x2, y2);
}
private void draw_horizontal_1(Graphics g, Pen pen, RectangleF bounds_rect)
{
float x1 = 1 + bounds_rect.X + this.LineWidth;
float y1 = bounds_rect.Y + this.cap_c;
float x2 = 1 + bounds_rect.X + this.line_w;
float y2 = bounds_rect.Y + this.cap_c;
g.DrawLine(pen, x1, y1, x2, y2);
}
private void draw_horizontal_2(Graphics g, Pen pen, RectangleF bounds_rect)
{
float x1 = 1 + bounds_rect.X + this.LineWidth;
float y1 = 2 + bounds_rect.Y + this.cap_c + this.line_h;
float x2 = 1 + bounds_rect.X + this.line_w;
float y2 = 2 + bounds_rect.Y + this.cap_c + this.line_h;
g.DrawLine(pen, x1, y1, x2, y2);
}
private void draw_horizontal_3(Graphics g, Pen pen, RectangleF bounds_rect)
{
float x1 = 1 + bounds_rect.X + this.LineWidth;
float y1 = 4 + bounds_rect.Y + this.cap_c + this.line_h + this.line_h;
float x2 = 1 + bounds_rect.X + this.line_w;
float y2 = 4 + bounds_rect.Y + this.cap_c + this.line_h + this.line_h;
g.DrawLine(pen, x1, y1, x2, y2);
}
private void draw_vertical_12(Graphics g, Pen pen, RectangleF bounds_rect)
{
float x1 = bounds_rect.X + this.cap_c + 2 + this.line_w;
float y1 = bounds_rect.Y + this.LineWidth + 1;
float x2 = bounds_rect.X + this.cap_c + 2 + this.line_w;
float y2 = bounds_rect.Y + this.line_h + 1;
g.DrawLine(pen, x1, y1, x2, y2);
}
private void draw_vertical_22(Graphics g, Pen pen, RectangleF bounds_rect)
{
float x1 = bounds_rect.X + this.cap_c + 2 + this.line_w;
float y1 = bounds_rect.Y + this.LineWidth + this.line_h + +3;
float x2 = bounds_rect.X + this.cap_c + 2 + this.line_w;
float y2 = bounds_rect.Y + this.line_h + this.line_h + 3;
g.DrawLine(pen, x1, y1, x2, y2);
}
#endregion
#region 绘制数字
private void draw_0(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
this.draw_vertical_11(g, highlight_pen, bounds_rect);
this.draw_vertical_21(g, highlight_pen, bounds_rect);
this.draw_horizontal_1(g, highlight_pen, bounds_rect);
if (this.ShadowShow)
this.draw_horizontal_2(g, shadow_pen, bounds_rect);
this.draw_horizontal_3(g, highlight_pen, bounds_rect);
this.draw_vertical_12(g, highlight_pen, bounds_rect);
this.draw_vertical_22(g, highlight_pen, bounds_rect);
}
private void draw_1(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
if (this.ShadowShow)
this.draw_vertical_11(g, shadow_pen, bounds_rect);
if (this.ShadowShow)
this.draw_vertical_21(g, shadow_pen, bounds_rect);
if (this.ShadowShow)
this.draw_horizontal_1(g, shadow_pen, bounds_rect);
if (this.ShadowShow)
this.draw_horizontal_2(g, shadow_pen, bounds_rect);
if (this.ShadowShow)
this.draw_horizontal_3(g, shadow_pen, bounds_rect);
this.draw_vertical_12(g, highlight_pen, bounds_rect);
this.draw_vertical_22(g, highlight_pen, bounds_rect);
}
private void draw_2(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
if (this.ShadowShow)
this.draw_vertical_11(g, shadow_pen, bounds_rect);
this.draw_vertical_21(g, highlight_pen, bounds_rect);
this.draw_horizontal_1(g, highlight_pen, bounds_rect);
this.draw_horizontal_2(g, highlight_pen, bounds_rect);
this.draw_horizontal_3(g, highlight_pen, bounds_rect);
this.draw_vertical_12(g, highlight_pen, bounds_rect);
if (this.ShadowShow)
this.draw_vertical_22(g, shadow_pen, bounds_rect);
}
private void draw_3(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
if (this.ShadowShow)
this.draw_vertical_11(g, shadow_pen, bounds_rect);
if (this.ShadowShow)
this.draw_vertical_21(g, shadow_pen, bounds_rect);
this.draw_horizontal_1(g, highlight_pen, bounds_rect);
this.draw_horizontal_2(g, highlight_pen, bounds_rect);
this.draw_horizontal_3(g, highlight_pen, bounds_rect);
this.draw_vertical_12(g, highlight_pen, bounds_rect);
this.draw_vertical_22(g, highlight_pen, bounds_rect);
}
private void draw_4(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
this.draw_vertical_11(g, highlight_pen, bounds_rect);
if (this.ShadowShow)
this.draw_vertical_21(g, shadow_pen, bounds_rect);
if (this.ShadowShow)
this.draw_horizontal_1(g, shadow_pen, bounds_rect);
this.draw_horizontal_2(g, highlight_pen, bounds_rect);
if (this.ShadowShow)
this.draw_horizontal_3(g, shadow_pen, bounds_rect);
this.draw_vertical_12(g, highlight_pen, bounds_rect);
this.draw_vertical_22(g, highlight_pen, bounds_rect);
}
private void draw_5(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
this.draw_vertical_11(g, highlight_pen, bounds_rect);
if (this.ShadowShow)
this.draw_vertical_21(g, shadow_pen, bounds_rect);
this.draw_horizontal_1(g, highlight_pen, bounds_rect);
this.draw_horizontal_2(g, highlight_pen, bounds_rect);
this.draw_horizontal_3(g, highlight_pen, bounds_rect);
if (this.ShadowShow)
this.draw_vertical_12(g, shadow_pen, bounds_rect);
this.draw_vertical_22(g, highlight_pen, bounds_rect);
}
private void draw_6(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
this.draw_vertical_11(g, highlight_pen, bounds_rect);
this.draw_vertical_21(g, highlight_pen, bounds_rect);
this.draw_horizontal_1(g, highlight_pen, bounds_rect);
this.draw_horizontal_2(g, highlight_pen, bounds_rect);
this.draw_horizontal_3(g, highlight_pen, bounds_rect);
if (this.ShadowShow)
this.draw_vertical_12(g, shadow_pen, bounds_rect);
this.draw_vertical_22(g, highlight_pen, bounds_rect);
}
private void draw_7(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
if (this.ShadowShow)
this.draw_vertical_11(g, shadow_pen, bounds_rect);
if (this.ShadowShow)
this.draw_vertical_21(g, shadow_pen, bounds_rect);
this.draw_horizontal_1(g, highlight_pen, bounds_rect);
if (this.ShadowShow)
this.draw_horizontal_2(g, shadow_pen, bounds_rect);
if (this.ShadowShow)
this.draw_horizontal_3(g, shadow_pen, bounds_rect);
this.draw_vertical_12(g, highlight_pen, bounds_rect);
this.draw_vertical_22(g, highlight_pen, bounds_rect);
}
private void draw_8(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
this.draw_vertical_11(g, highlight_pen, bounds_rect);
this.draw_vertical_21(g, highlight_pen, bounds_rect);
this.draw_horizontal_1(g, highlight_pen, bounds_rect);
this.draw_horizontal_2(g, highlight_pen, bounds_rect);
this.draw_horizontal_3(g, highlight_pen, bounds_rect);
this.draw_vertical_12(g, highlight_pen, bounds_rect);
this.draw_vertical_22(g, highlight_pen, bounds_rect);
}
private void draw_9(Graphics g, Pen highlight_pen, RectangleF bounds_rect, Pen shadow_pen)
{
this.draw_vertical_11(g, highlight_pen, bounds_rect);
if (this.ShadowShow)
this.draw_vertical_21(g, shadow_pen, bounds_rect);
this.draw_horizontal_1(g, highlight_pen, bounds_rect);
this.draw_horizontal_2(g, highlight_pen, bounds_rect);
this.draw_horizontal_3(g, highlight_pen, bounds_rect);
this.draw_vertical_12(g, highlight_pen, bounds_rect);
this.draw_vertical_22(g, highlight_pen, bounds_rect);
}
private void draw_split(Graphics g, SolidBrush highlight_sb, RectangleF bounds_rect)
{
RectangleF top_rect = new RectangleF(bounds_rect.X + (bounds_rect.Width - this.LineWidth) / 2, bounds_rect.Y + (bounds_rect.Height / 2 - this.LineWidth) / 2, this.LineWidth, this.LineWidth);
RectangleF bottom_rect = new RectangleF(bounds_rect.X + (bounds_rect.Width - this.LineWidth) / 2, bounds_rect.Y + bounds_rect.Height / 2 + (bounds_rect.Height / 2 - this.LineWidth) / 2, this.LineWidth, this.LineWidth);
g.FillEllipse(highlight_sb, top_rect);
g.FillEllipse(highlight_sb, bottom_rect);
}
///
/// 绘制数字
///
///
/// 数字高亮颜色
/// 数字的rect
/// 数字阴影颜色
/// 数字
private void draw_num(Grap