标签:src http control end normal count date() anti 控件
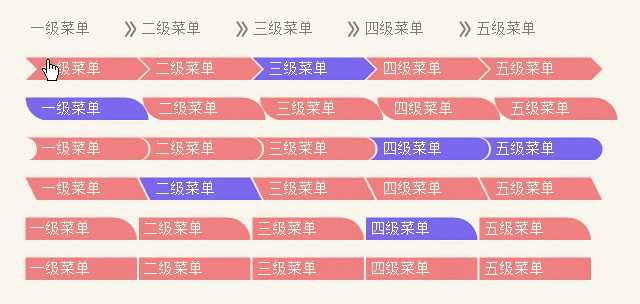
///
/// 导航导航栏控件
///
[ToolboxItem(true)]
[DefaultProperty("Items")]
[DefaultEvent("NavigationItemClick")]
[Description("导航导航栏控件")]
public partial class NavigationBarExt : Control
{
public delegate void EventHandler(object sender, NavigationItemEventArgs e);
private event EventHandler navigationItemClick;
///
/// 导航选项单击事件
///
[Description("导航选项单击事件")]
public event EventHandler NavigationItemClick
{
add { this.navigationItemClick += value; }
remove { this.navigationItemClick -= value; }
}
#region
private bool borderShow = false;
///
///是否显示边框
///
[DefaultValue(false)]
[Description("是否显示边框")]
public bool BorderShow
{
get { return this.borderShow; }
set
{
if (this.borderShow == value)
return;
this.borderShow = value;
this.InitializeItemsSize();
this.Invalidate();
}
}
private ButtonBorderStyle borderStyle = ButtonBorderStyle.Solid;
///
///边框样式
///
[DefaultValue(ButtonBorderStyle.Solid)]
[Description("边框样式")]
public ButtonBorderStyle BorderStyle
{
get { return this.borderStyle; }
set
{
if (this.borderStyle == value)
return;
this.borderStyle = value;
this.InitializeItemsSize();
this.Invalidate();
}
}
private int borderWidth = 0;
///
///边框宽度
///
[DefaultValue(0)]
[Description("边框宽度")]
public int BorderWidth
{
get { return this.borderWidth; }
set
{
if (this.borderWidth == value)
return;
this.borderWidth = value;
this.InitializeItemsSize();
this.Invalidate();
}
}
private int itemMinWidth = 0;
///
///选项最小宽度
///
[DefaultValue(0)]
[Description("选项最小宽度")]
public int ItemMinWidth
{
get { return this.itemMinWidth; }
set
{
if (this.itemMinWidth == value || value 0)
return;
this.itemMinWidth = value;
this.InitializeItemsSize();
this.Invalidate();
}
}
private int itemMaxWidth = 0;
///
///选项最大宽度
///
[DefaultValue(0)]
[Description("选项最大宽度")]
public int ItemMaxWidth
{
get { return this.itemMaxWidth; }
set
{
if (this.itemMaxWidth == value || value this.ItemMinWidth)
return;
this.itemMaxWidth = value;
this.InitializeItemsSize();
this.Invalidate();
}
}
private Color borderColor = Color.Gray;
///
/// 边框颜色
///
[DefaultValue(typeof(Color), "Gray")]
[Description("边框颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color BorderColor
{
get { return this.borderColor; }
set
{
if (this.borderColor == value)
return;
this.borderColor = value;
this.Invalidate();
}
}
private Font textFont = new Font("宋体", 11);
///
/// 文本字体
///
[DefaultValue(typeof(Font), "宋体, 11pt")]
[Description("文本字体")]
public Font TextFont
{
get { return this.textFont; }
set
{
if (this.textFont == value)
return;
this.textFont = value;
this.Invalidate();
}
}
private Color arrowsColor = Color.Gray;
///
/// 箭头颜色
///
[DefaultValue(typeof(Color), "Gray")]
[Description("箭头颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color ArrowsColor
{
get { return this.arrowsColor; }
set
{
if (this.arrowsColor == value)
return;
this.arrowsColor = value;
this.Invalidate();
}
}
private Color textColor = Color.White;
///
/// 文本颜色
///
[DefaultValue(typeof(Color), "White")]
[Description("文本颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color TextColor
{
get { return this.textColor; }
set
{
if (this.textColor == value)
return;
this.textColor = value;
this.Invalidate();
}
}
private Color textBackColor = Color.LightCoral;
///
/// 文本背景颜色
///
[DefaultValue(typeof(Color), "LightCoral")]
[Description("文本背景颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color TextBackColor
{
get { return this.textBackColor; }
set
{
if (this.textBackColor == value)
return;
this.textBackColor = value;
this.Invalidate();
}
}
private Color textEnterColor = Color.White;
///
/// 鼠标进入文本颜色
///
[DefaultValue(typeof(Color), "White")]
[Description("鼠标进入文本颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color TextEnterColor
{
get { return this.textEnterColor; }
set
{
if (this.textEnterColor == value)
return;
this.textEnterColor = value;
this.Invalidate();
}
}
private Color textEnterBackColor = Color.IndianRed;
///
/// 鼠标进入文本背景颜色
///
[DefaultValue(typeof(Color), "IndianRed")]
[Description("鼠标进入文本背景颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color TextEnterBackColor
{
get { return this.textEnterBackColor; }
set
{
if (this.textEnterBackColor == value)
return;
this.textEnterBackColor = value;
this.Invalidate();
}
}
private Color textSelectedColor = Color.White;
///
/// 文本选中颜色
///
[DefaultValue(typeof(Color), "White")]
[Description("文本选中颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color TextSelectedColor
{
get { return this.textSelectedColor; }
set
{
if (this.textSelectedColor == value)
return;
this.textSelectedColor = value;
this.Invalidate();
}
}
private Color textSelectedBackColor = Color.MediumSlateBlue;
///
/// 文本选中背景颜色
///
[DefaultValue(typeof(Color), "MediumSlateBlue")]
[Description("文本选中背景颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color TextSelectedBackColor
{
get { return this.textSelectedBackColor; }
set
{
if (this.textSelectedBackColor == value)
return;
this.textSelectedBackColor = value;
this.Invalidate();
}
}
private StyleType style = StyleType.Normal;
///
/// 导航样式
///
[DefaultValue(StyleType.Normal)]
[Description("导航样式")]
public StyleType Style
{
get { return this.style; }
set
{
if (this.style == value)
return;
this.style = value;
this.InitializeItemsSize();
this.Invalidate();
}
}
private NavigationItemCollection items;
///
/// 导航选项集合
///
[DefaultValue(null)]
[Description("导航选项集合")]
[DesignerSerializationVisibility(DesignerSerializationVisibility.Content)]
public NavigationItemCollection Items
{
get
{
if (this.items == null)
this.items = new NavigationItemCollection(this);
return this.items;
}
}
protected override Size DefaultSize
{
get
{
return new Size(300, 23);
}
}
#endregion
public NavigationBarExt()
{
SetStyle(ControlStyles.UserPaint, true);
SetStyle(ControlStyles.AllPaintingInWmPaint, true);
SetStyle(ControlStyles.OptimizedDoubleBuffer, true);
SetStyle(ControlStyles.ResizeRedraw, true);
SetStyle(ControlStyles.SupportsTransparentBackColor, true);
InitializeComponent();
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
Graphics g = e.Graphics;
g.SmoothingMode = SmoothingMode.AntiAlias;
g.TextRenderingHint = System.Drawing.Text.TextRenderingHint.SingleBitPerPixelGridFit;
Rectangle rect = e.ClipRectangle;
if (this.BorderShow)
{
ControlPaint.DrawBorder(g, rect, this.BorderColor, this.BorderWidth, this.BorderStyle, this.BorderColor, this.BorderWidth, this.BorderStyle, this.BorderColor, this.BorderWidth, this.BorderStyle, this.BorderColor, this.BorderWidth, this.BorderStyle);
}
for (int i = 0; i this.Items.Count; i++)
{
Color color = this.Items[i].TextColor == Color.Empty ? this.TextColor : this.Items[i].TextColor;
Color backcolor = this.Items[i].TextBackColor == Color.Empty ? this.TextBackColor : this.Items[i].TextBackColor;
if (this.Items[i].MoveStatus == ItemType.Enter)
{
color = this.Items[i].TextEnterColor == Color.Empty ? this.TextEnterColor : this.Items[i].TextEnterColor;
backcolor = this.Items[i].TextEnterBackColor == Color.Empty ? this.TextEnterBackColor : this.Items[i].TextEnterBackColor;
}
else if (this.Items[i].Selected)
{
color = this.Items[i].TextSelectedColor == Color.Empty ? this.TextSelectedColor : this.Items[i].TextSelectedColor;
backcolor = this.Items[i].TextSelectedBackColor == Color.Empty ? this.TextSelectedBackColor : this.Items[i].TextSelectedBackColor;
}
SolidBrush back_sb = new SolidBrush(backcolor);
if (this.Style == StyleType.Normal)
{
g.FillRectangle(back_sb, this.Items[i].TextRectF);
TextRenderer.DrawText(g, this.Items[i].Text, this.TextFont, new Rectangle((int)this.Items[i].TextRectF.X, (int)this.Items[i].TextRectF.Y, (int)this.Items[i].TextRectF.Width, (int)this.Items[i].TextRectF.Height), color, TextFormatFlags.LeftAndRightPadding | TextFormatFlags.VerticalCenter);
if (i this.Items.Count - 1)
{
float w = this.Items[i].ArrowsRectF.Width - 4;
Pen arrows_pen = new Pen(this.ArrowsColor, 2);
g.DrawLine(arrows_pen, this.Items[i].ArrowsRectF.X, this.Items[i].ArrowsRectF.Y, this.Items[i].ArrowsRectF.X + w / 6 * 5, this.Items[i].ArrowsRectF.Y + this.Items[i].ArrowsRectF.Height / 2);
g.DrawLine(arrows_pen, this.Items[i].ArrowsRectF.X + w / 6 * 5, this.Items[i].ArrowsRectF.Y + this.Items[i].ArrowsRectF.Height / 2, this.Items[i].ArrowsRectF.X, this.Items[i].ArrowsRectF.Y + this.Items[i].ArrowsRectF.Height);
g.DrawLine(arrows_pen, this.Items[i].ArrowsRectF.X + w / 6 + 4, this.Items[i].ArrowsRectF.Y, this.Items[i].ArrowsRectF.X + w + 4, this.Items[i].ArrowsRectF.Y + this.Items[i].ArrowsRectF.Height / 2);
g.DrawLine(arrows_pen, this.Items[i].ArrowsRectF.X + w + 4, this.Items[i].ArrowsRectF.Y + this.Items[i].ArrowsRectF.Height / 2, this.Items[i].ArrowsRectF.X + w / 6 + 4, this.Items[i].ArrowsRectF.Y + this.Items[i].ArrowsRectF.Height);
arrows_pen.Dispose();
}
}
else if (this.Style == StyleType.Parallelogram)
{
GraphicsPath back_gp = new GraphicsPath();
back_gp.AddPolygon(new PointF[] {
new PointF(this.Items[i].TextRectF.X, this.Items[i].TextRectF.Y),
new PointF(this.Items[i].TextRectF.Right - this.Items[i].TextRectF.Height / 2, this.Items[i].TextRectF.Y),
new PointF(this.Items[i].TextRectF.Right , this.Items[i].TextRectF.Bottom),
new PointF(this.Items[i].TextRectF.X + this.Items[i].TextRectF.Height / 2, this.Items[i].TextRectF.Bottom)});
g.FillPath(back_sb, back_gp);
back_gp.Dispose();
TextRenderer.DrawText(g, this.Items[i].Text, this.TextFont, new Rectangle((int)(this.Items[i].TextRectF.X + this.Items[i].TextRectF.Height / 2), (int)this.Items[i].TextRectF.Y, (int)this.Items[i].TextRectF.Width, (int)this.Items[i].TextRectF.Height), color, TextFormatFlags.LeftAndRightPadding | TextFormatFlags.VerticalCenter);
}
else if (this.Style == StyleType.Arrows)
{
GraphicsPath back_gp = new GraphicsPath();
back_gp.AddPolygon(new PointF[] {
new PointF(this.Items[i].TextRectF.X, this.Items[i].TextRectF.Y),
new PointF(this.Items[i].TextRectF.Right- this.Items[i].TextRectF.Height / 2, this.Items[i].TextRectF.Y),
new PointF(this.Items[i].TextRectF.Right, this.Items[i].TextRectF.Y+this.Items[i].TextRectF.Height / 2),
new PointF(this.Items[i].TextRectF.Right- this.Items[i].TextRectF.Height / 2, this.Items[i].TextRectF.Bottom),
new PointF(this.Items[i].TextRectF.X , this.Items[i].TextRectF.Bottom),
new PointF(this.Items[i].TextRectF.X+this.Items[i].TextRectF.Height / 2 , this.Items[i].TextRectF.Y+this.Items[i].TextRectF.Height / 2)});
g.FillPath(back_sb, back_gp);
back_gp.Dispose();
TextRenderer.DrawText(g, this.Items[i].Text, this.TextFont, new Rectangle((int)(this.Items[i].TextRectF.X + this.Items[i].TextRectF.Height / 2), (int)this.Items[i].TextRectF.Y, (int)this.Items[i].TextRectF.Width, (int)this.Items[i].TextRectF.Height), color, TextFormatFlags.LeftAndRightPadding | TextFormatFlags.VerticalCenter);
}
else if (this.Style == StyleType.RoundCap)
{
GraphicsPath back_gp = new GraphicsPath();
back_gp.AddArc(new RectangleF(this.Items[i].TextRectF.X - this.Items[i].TextRectF.Height / 2, this.Items[i].TextRectF.Y, this.Items[i].TextRectF.Height, this.Items[i].TextRectF.Height), 270, 180);
back_gp.Reverse();
back_gp.AddArc(new RectangleF(this.Items[i].TextRectF.Right - this.Items[i].TextRectF.Height, this.Items[i].TextRectF.Y, this.Items[i].TextRectF.Height, this.Items[i].TextRectF.Height), 270, 180);
g.FillPath(back_sb, back_gp);
back_gp.Dispose();
TextRenderer.DrawText(g, this.Items[i].Text, this.TextFont, new Rectangle((int)(this.Items[i].TextRectF.X + this.Items[i].TextRectF.Height / 2), (int)this.Items[i].TextRectF.Y, (int)this.Items[i].TextRectF.Width, (int)this.Items[i].TextRectF.Height), color, TextFormatFlags.LeftAndRightPadding | TextFormatFlags.VerticalCenter);
}
else if (this.Style == StyleType.Quadrangle)
{
g.FillRectangle(back_sb, this.Items[i].TextRectF);
TextRenderer.DrawText(g, this.Items[i].Text, this.TextFont, new Rectangle((int)this.Items[i].TextRectF.X, (int)this.Items[i].TextRectF.Y, (int)this.Items[i].TextRectF.Width, (int)this.Items[i].TextRectF.Height), color, TextFormatFlags.LeftAndRightPadding | TextFormatFlags.VerticalCenter);
}
else if (this.Style == StyleType.Circular)
{
GraphicsPath back_gp = new GraphicsPath();
back_gp.AddArc(new RectangleF(this.Items[i].TextRectF.Right - this.Items[i].TextRectF.Height * 2, this.Items[i].TextRectF.Y, this.Items[i].TextRectF.Height * 2, this.Items[i].TextRectF.Height * 2), 270, 90);
back_gp.AddLine(this.Items[i].TextRectF.X, this.Items[i].TextRectF.Bottom, this.Items[i].TextRectF.X, this.Items[i].TextRectF.Y);
g.FillPath(back_sb, back_gp);
back_gp.Dispose();
TextRenderer.DrawText(g, this.Items[i].Text, this.TextFont, new Rectangle((int)this.Items[i].TextRectF.X, (int)this.Items[i].TextRectF.Y, (int)this.Items[i].TextRectF.Width, (int)this.Items[i].TextRectF.Height), color, TextFormatFlags.LeftAndRightPadding | TextFormatFlags.VerticalCenter);
}
else if (this.Style == StyleType.Leaf)
{
GraphicsPath back_gp = new GraphicsPath();
back_gp.AddArc(new RectangleF(this.Items[i].TextRectF.Right - this.Items[i].TextRectF.Height * 2, this.Items[i].TextRectF.Y, this.Items[i].TextRectF.Height * 2, this.Items[i].TextRectF.Height * 2), 270, 90);
back_gp.AddArc(new RectangleF(this.Items[i].TextRectF.X, -this.Items[i].TextRectF.Height, this.Items[i].TextRectF.Height * 2, this.Items[i].TextRectF.Height * 2), 90, 90);
g.FillPath(back_sb, back_gp);
back_gp.Dispose();
TextRenderer.DrawText(g, this.Items[i].Text, this.TextFont, new Rectangle((int)(this.Items[i].TextRectF.X + this.Items[i].TextRectF.Height / 2), (int)this.Items[i].TextRectF.Y, (int)this.Items[i].TextRectF.Width, (int)this.Items[i].TextRectF.Height), color, TextFormatFlags.LeftAndRightPadding | TextFormatFlags.VerticalCenter);
}
back_sb.Dispose();
}
}
///
/// 初始化导航选项rect
///
private void InitializeItemsSize()
{
Graphics g = this.CreateGraphics();
RectangleF rect = g.VisibleClipBounds;
float b = this.BorderShow ? this.BorderWidth : 0;
float start_x = b;
float arrows_h = rect.Height - b * 2;
float arrows_w = arrows_h / 2;
for (int i = 0; i this.Items.Count; i++)
{
Size text_size = TextRenderer.MeasureText(g, this.Items[i].Text, this.TextFont, new Size(0, 0), TextFormatFlags.LeftAndRightPadding);
if (this.ItemMinWidth != 0)
{
text_size.Width = Math.Max(text_size.Width, this.ItemMinWidth);
}
if (this.ItemMaxWidth != 0)
{
text_size.Width = Math.Min(text_size.Width, this.ItemMaxWidth);
}
if (this.Style == StyleType.Normal)
{
this.Items[i].TextRectF = new RectangleF(start_x, b, text_size.Width, arrows_h);
this.Items[i].ArrowsRectF = new RectangleF(this.Items[i].TextRectF.Right, (rect.Height - text_size.Height) / 2, text_size.Height / 3 * 2, text_size.Height);
start_x += text_size.Width + arrows_w;
}
else if (this.Style == StyleType.Parallelogram || this.Style == StyleType.Arrows || this.Style == StyleType.RoundCap)
{
this.Items[i].TextRectF = new RectangleF(start_x, b, text_size.Width + arrows_w * 2, arrows_h);
start_x += text_size.Width + arrows_w + 2;
}
else if (this.Style == StyleType.Quadrangle || this.Style == StyleType.Circular)
{
this.Items[i].TextRectF = new RectangleF(start_x, b, text_size.Width + arrows_h / 2, arrows_h);
start_x += text_size.Width + arrows_h / 2 + 2;
}
else if (this.Style == StyleType.Quadrangle || this.Style == StyleType.Leaf)
{
this.Items[i].TextRectF = new RectangleF(start_x, b, text_size.Width + arrows_h, arrows_h);
start_x += text_size.Width + arrows_h - arrows_w / 2;
}
}
g.Dispose();
this.Invalidate();
}
protected override void OnResize(EventArgs e)
{
base.OnResize(e);
this.InitializeItemsSize();
}
protected override void OnClick(EventArgs e)
{
base.OnClick(e);
Point point = this.PointToClient(Control.MousePosition);
if (this.navigationItemClick != null)
{
for (int i = 0; i this.Items.Count; i++)
{
if (this.Items[i].TextRectF.Contains(point))
{
this.navigationItemClick(this, new NavigationItemEventArgs() { Item = this.Items[i] });
break;
}
}
}
}
protected override void OnMouseMove(MouseEventArgs e)
{
base.OnMouseMove(e);
bool isenter = false;
for (int i = 0; i this.Items.Count; i++)
{
if (this.Items[i].TextRectF.Contains(e.Location))
{
this.Items[i].MoveStatus = ItemType.Enter;
isenter = true;
}
else
{
this.Items[i].MoveStatus = this.Items[i].Selected ? ItemType.Selected : ItemType.Normal;
}
}
if (isenter)
{
if (this.Cursor != Cursors.Hand)
{
this.Cursor = Cursors.Hand;
}
}
else
{
this.Cursor = Cursors.Default;
}
this.Invalidate();
}
protected override void OnMouseLeave(EventArgs e)
{
base.OnMouseLeave(e);
this.Cursor = Cursors.Default;
for (int i = 0; i this.Items.Count; i++)
{
this.Items[i].MoveStatus = this.Items[i].Selected ? ItemType.Selected : ItemType.Normal;
}
this.Invalidate();
}
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
///
/// 导航选项集合
///
[Description("导航选项集合")]
[Editor(typeof(CollectionEditorExt), typeof(UITypeEditor))]
public sealed class NavigationItemCollection : IList, ICollection, IEnumerable
{
private ArrayList navigationItemList = new ArrayList();
private NavigationBarExt owner;
public NavigationItemCollection(NavigationBarExt owner)
{
this.owner = owner;
}
#region IEnumerable
public IEnumerator GetEnumerator()
{
NavigationItem[] listArray = new NavigationItem[this.navigationItemList.Count];
for (int index = 0; index index)
listArray[index] = (NavigationItem)this.navigationItemList[index];
return listArray.GetEnumerator();
}
#endregion
#region ICollection
public void CopyTo(Array array, int index)
{
for (int i = 0; i this.Count; i++)
array.SetValue(this.navigationItemList[i], i + index);
}
public int Count
{
get
{
return this.navigationItemList.Count;
}
}
public bool IsSynchronized
{
get
{
return false;
}
}
public object SyncRoot
{
get
{
return (object)this;
}
}
#endregion
#region IList
public int Add(object value)
{
NavigationItem navigationItem = (NavigationItem)value;
this.navigationItemList.Add(navigationItem);
this.owner.InitializeItemsSize();
return this.Count - 1;
}
public void Clear()
{
this.navigationItemList.Clear();
this.owner.InitializeItemsSize();
}
public bool Contains(object value)
{
return this.IndexOf(value) != -1;
}
public int IndexOf(object value)
{
return this.navigationItemList.IndexOf(value);
}
public void Insert(int index, object value)
{
throw new NotImplementedException();
}
public bool IsFixedSize
{
get { return false; }
}
public bool IsReadOnly
{
get { return false; }
}
public void Remove(object value)
{
if (!(value is NavigationItem))
return;
this.navigationItemList.Remove((NavigationItem)value);
this.owner.InitializeItemsSize();
}
public void RemoveAt(int index)
{
this.navigationItemList.RemoveAt(index);
this.owner.InitializeItemsSize();
}
public NavigationItem this[int index]
{
get
{
return (NavigationItem)this.navigationItemList[index];
}
set
{
this.navigationItemList[index] = (NavigationItem)value;
this.owner.InitializeItemsSize();
}
}
object IList.this[int index]
{
get
{
return (object)this.navigationItemList[index];
}
set
{
this.navigationItemList[index] = (NavigationItem)value;
this.owner.InitializeItemsSize();
}
}
#endregion
}
///
/// 实际坐标
///
[Description("实际坐标")]
public class NavigationItem
{
private string text;
///
/// 文本
///
[DefaultValue("")]
[Description("文本")]
public string Text
{
get { return this.text; }
set
{
if (this.text == value)
return;
this.text = value;
}
}
private Color textColor = Color.Empty;
///
/// 文本颜色
///
[DefaultValue(typeof(Color), "Empty")]
[Description("文本颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color TextColor
{
get { return this.textColor; }
set
{
if (this.textColor == value)
return;
this.textColor = value;
}
}
private Color textBackColor = Color.Empty;
///
/// 文本背景颜色
///
[DefaultValue(typeof(Color), "Empty")]
[Description("文本背景颜色")]
[Editor(typeof(ColorEditorExt), typeof(System.Drawing.Design.UITypeEditor))]
public Color TextBackColor
{
get { return this.textBackColor; }
set
{
if (this.textBackColor == value)
return;
this.textBackColor = value;
}
}
private Color textEnterColor = Color.Empty;
/// &l