winfrom窗体加载控制台程序,可以自定义输出语句颜色
标签:xxxxx get port isp 一个 white 地方 gray 交互
winfrom窗体加载控制台程序,可以自定方输出语句颜色,如下图所示
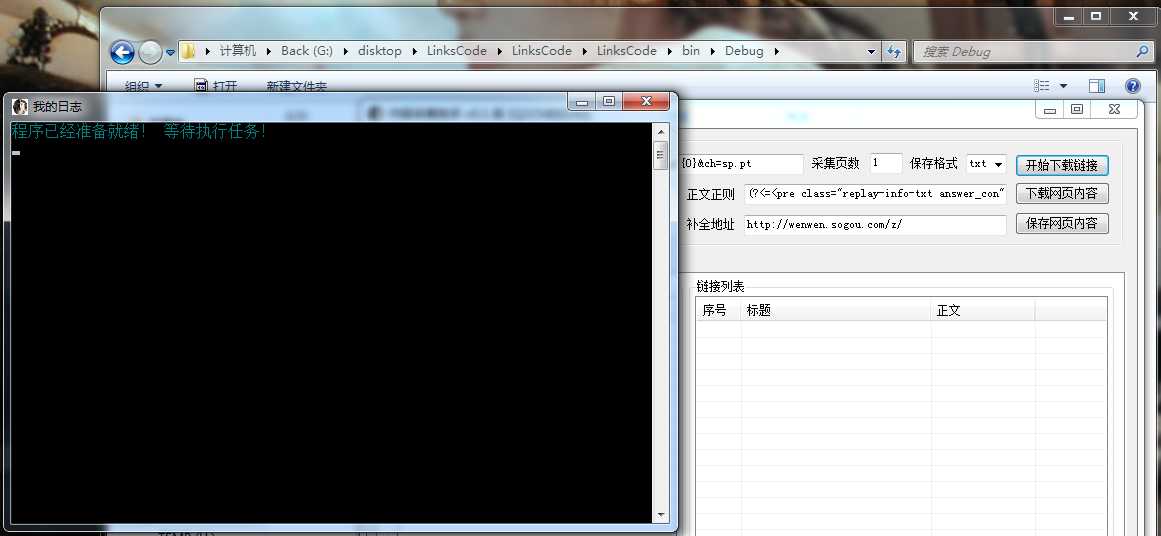
怎么实现的此功能,网上有大把的方法,我这里已经把方法打包成了一个类,只需要引用调用就可以使用了,写的比较粗糙,如有发现需要改进的地方欢迎提出!
至于使用方法很简单,把这个类复制到解决方案中,在引用 LLog , 另外在 程序初始化下面加上 XLog.AllocConsole(); 就可以在想使用的地方调用方法 XLog.Logx() 使用了,
如图所示:
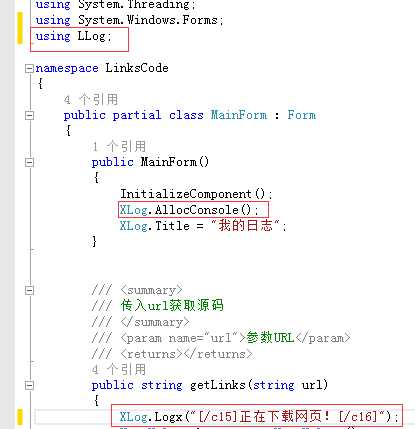
注意:图片中显示 [/c15] xxxxxx[/c16] 必须成对出现才不会出现输出颜色覆盖下一行内容, 此方法效率略低,水平有限还没想出高效方法,如想某行只输出某种颜色必须在语句后面加上 [/c16] 结尾 如:[/c1] 我是内容 [/c16]
1 using System;
2 using System.Collections.Generic;
3 using System.Linq;
4 using System.Runtime.InteropServices;
5 using System.Text;
6
7 namespace LLog
8 {
9 ///
10 /// 与控制台交互
11 ///
12 static class XLog
13 {
14
15 [DllImport("kernel32.dll")]
16 public static extern bool AllocConsole();
17 [DllImport("kernel32.dll")]
18 public static extern bool FreeConsole();
19
20 ///
21 /// 修改控制台标题
22 ///
23 public static string Title
24 {
25 get
26 {
27 string result;
28 try
29 {
30 result = Console.Title;
31 }
32 catch
33 {
34 result = "";
35 }
36 return result;
37 }
38 set
39 {
40 Console.Title = value;
41 }
42 }
43
44
45 ///
46 /// 调用输出日志方法从c1-c15 共15种颜色
47 ///
48 /// [c1]蓝色 [c2]青色(蓝绿色) [c3]藏蓝色 [c4]深紫色(深蓝绿色) [c5]深灰色 [c6]深绿色 [c7]深紫红色 [c8]深红色 [c9]深黄色 [c10]灰色 [c11]绿色 [c12]紫红色 [c13]红色 [c14]白色 [c15]黄色 [c16]默认灰色
49 public static void Logx_Console(string code)
50 {
51 //①②③④⑤⑥⑦⑧⑨⑩⑾⑿⒀⒁⒂
52 string str = code;
53 string output = str.Replace("[/c1]", "①").Replace("[/c2]", "②").Replace("[/c3]", "③").Replace("[/c4]", "④").Replace("[/c5]", "⑤").Replace("[/c6]", "⑥").Replace("[/c7]", "⑦").Replace("[/c8]", "⑧").Replace("[/c9]", "⑨").Replace("[/c10]", "⑩").Replace("[/c11]", "⑾").Replace("[/c12]", "⑿").Replace("[/c13]", "⒀").Replace("[/c14]", "⒁").Replace("[/c15]", "⒂").Replace("[/c16]","⒃");
54 string display = "";
55 foreach (char c in output)
56 {
57 if (c == ‘①‘)
58 {
59 Console.ForegroundColor = ConsoleColor.Blue;
60 }
61
62 if (c == ‘②‘)
63 {
64 Console.ForegroundColor = ConsoleColor.Cyan;
65 }
66
67 if (c == ‘③‘)
68 {
69 Console.ForegroundColor = ConsoleColor.DarkBlue;
70 }
71 if (c == ‘④‘)
72 {
73 Console.ForegroundColor = ConsoleColor.DarkCyan;
74 }
75
76 if (c == ‘⑤‘)
77 {
78 Console.ForegroundColor = ConsoleColor.DarkGray;
79 }
80
81 if (c == ‘⑥‘)
82 {
83 Console.ForegroundColor = ConsoleColor.DarkGreen;
84 }
85
86 if (c == ‘⑦‘)
87 {
88 Console.ForegroundColor = ConsoleColor.DarkMagenta;
89 }
90
91 if (c == ‘⑧‘)
92 {
93 Console.ForegroundColor = ConsoleColor.DarkRed;
94 }
95
96 if (c == ‘⑨‘)
97 {
98 Console.ForegroundColor = ConsoleColor.DarkYellow;
99 }
100
101 if (c == ‘⑩‘)
102 {
103 Console.ForegroundColor = ConsoleColor.Gray;
104 }
105
106 if (c == ‘⑾‘)
107 {
108 Console.ForegroundColor = ConsoleColor.Green;
109 }
110
111 if (c == ‘⑿‘)
112 {
113 Console.ForegroundColor = ConsoleColor.Magenta;
114 }
115
116 if (c == ‘⒀‘)
117 {
118 Console.ForegroundColor = ConsoleColor.Red;
119 }
120
121 if (c == ‘⒁‘)
122 {
123 Console.ForegroundColor = ConsoleColor.White;
124 }
125
126 if (c == ‘⒂‘)
127 {
128 Console.ForegroundColor = ConsoleColor.Yellow;
129 }
130
131 if (c == ‘⒃‘)
132 {
133 Console.ForegroundColor = ConsoleColor.Gray;
134 }
135
136 display = c.ToString();
137 display = display.Replace("①", "").Replace("②", "").Replace("③", "").Replace("④", "").Replace("⑤", "").Replace("⑥", "").Replace("⑦", "").Replace("⑧", "").Replace("⑨", "").Replace("⑩", "").Replace("⑾", "").Replace("⑿", "").Replace("⒀", "").Replace("⒁", "").Replace("⒂", "").Replace("⒃", "");
138 Console.Write(display);
139 }
140 }
141
142 ///
143 /// 传入字符跟指定的颜色标签
144 ///
145 /// [c1]蓝色 [c2]青色(蓝绿色) [c3]藏蓝色 [c4]深紫色(深蓝绿色) [c5]深灰色 [c6]深绿色 [c7]深紫红色 [c8]深红色 [c9]深黄色 [c10]灰色 [c11]绿色 [c12]紫红色 [c13]红色 [c14]白色 [c15]黄色 [c16]默认灰色
146 public static void Logx(string str)
147 {
148 XLog.Logx_Console(str + "\r\n");
149 }
150 }
151 }
winfrom窗体加载控制台程序,可以自定义输出语句颜色
标签:xxxxx get port isp 一个 white 地方 gray 交互
原文地址:http://www.cnblogs.com/ncle/p/7745032.html
评论