C语言单项链表
标签:roo 直接 val star move getchar 记录 res eof
记录下来,以后用到了直接照抄。
代码
#include
#include
typedef struct node {
struct node *next;
int value;
} *SingleList;
int value_compare(struct node *a, int i)
{
return (a->value - i);
}
struct node *sl_find(struct node **rootptr, int v, int (*compare)(struct node *, int))
{
while(*rootptr)
{
if (0 == compare(*rootptr, v))
{
return *rootptr;
}
rootptr = &(*rootptr)->next;
}
return NULL;
}
int sl_remove(struct node **rootptr, struct node *which)
{
while(*rootptr)
{
if (*rootptr == which)
{
struct node *t = *rootptr;
*rootptr = t->next;
return 1;
}
rootptr = &(*rootptr)->next;
}
return 0;
}
int sl_append(struct node **rootptr, struct node *n)
{
while(*rootptr)
rootptr = &(*rootptr)->next;
*rootptr = n;
return 1;
}
struct node *sl_fetch(struct node **rootptr)
{
struct node *t;
t = *rootptr;
if (NULL != t)
*rootptr = t->next;
else
*rootptr = NULL;
return t;
}
void single_list_test(void)
{
const int TOTAL = 10;
int i;
struct node *root = NULL;
struct node *temp;
struct node **rootptr;
printf("single list test start\n");
printf("---------------------\n");
for (i=0; i)
{
temp = (struct node *)malloc(sizeof(struct node));
temp->value = i;
temp->next = NULL;
printf("append node %d\n", i);
sl_append(&root, temp);
}
printf("---------------------\n");
if (NULL != (temp = sl_fetch(&root)))
{
printf("fetch node %d\n", temp->value);
free(temp);
}
if (NULL != (temp = sl_fetch(&root)))
{
printf("fetch node %d\n", temp->value);
free(temp);
}
if (NULL != (temp = sl_find(&root, 2, value_compare)))
{
printf("delete node %d\n", temp->value);
sl_remove(&root,temp);
free(temp);
}
if (NULL != (temp = sl_find(&root, 12, value_compare)))
{
printf("delete node %d\n", temp->value);
sl_remove(&root,temp);
free(temp);
}
if (NULL != (temp = sl_find(&root, 8, value_compare)))
{
printf("delete node %d\n", temp->value);
sl_remove(&root,temp);
free(temp);
}
if (NULL != (temp = sl_find(&root, 5, value_compare)))
{
printf("delete node %d\n", temp->value);
sl_remove(&root,temp);
free(temp);
}
printf("---------------------\n");
rootptr = &root;
while(*rootptr)
{
temp = *rootptr;
*rootptr = temp->next;
printf("free %d\n", temp->value);
free(temp);
}
printf("---------------------\n");
printf("single list test finish\n\n");
}
int main(void)
{
single_list_test();
printf("press ENTER key to exit!\n");
getchar();
return 0;
}
截图
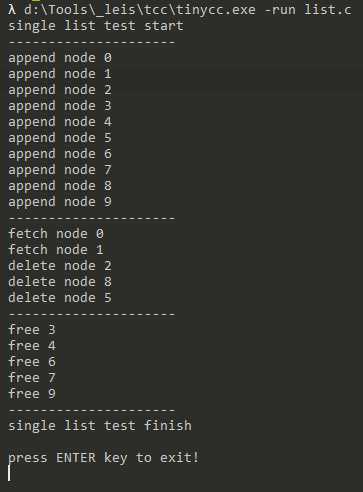
C语言单项链表
标签:roo 直接 val star move getchar 记录 res eof
原文地址:https://www.cnblogs.com/ssdq/p/13186719.html
文章来自:
搜素材网的
编程语言模块,转载请注明文章出处。
文章标题:
C语言单项链表
文章链接:http://soscw.com/index.php/essay/83520.html
评论