标签:listt 准备 使用 ble 数据源 index summary package tran
参考:https://www.cnblogs.com/caofangsheng/p/6149843.html
简介:Epplus是一个使用Open Office XML(Xlsx)文件格式,能读写Excel 2007/2010文件的开源组件
功效:支持对excel文档的汇入汇出,图表(excel自带的图表基本都可以实现)的列印
源代码下载:https://github.com/caofangsheng93/ExcelExportInMvc
导出思路:
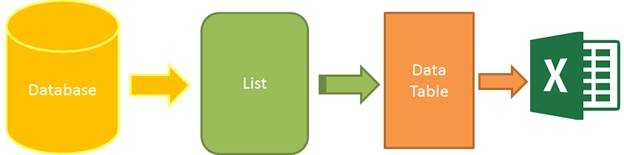
准备:
1.创建对象
public class Student
{
public int ID { get; set; }
public string Name { get; set; }
public string Sex { get; set; }
public int Age { get; set;
public string Email { get; set; }
}
1.创建Excel帮助类
///
/// Excel导出帮助类
///
public class ExcelExportHelper
{
public static string ExcelContentType
{
get
{
return "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet";
}
}
///
/// List转DataTable
///
///
///
///
public static DataTable ListToDataTable(List data)
{
PropertyDescriptorCollection properties = TypeDescriptor.GetProperties(typeof(T));
DataTable dataTable = new DataTable();
for (int i = 0; i )
{
PropertyDescriptor property = properties[i];
dataTable.Columns.Add(property.Name, Nullable.GetUnderlyingType(property.PropertyType) ?? property.PropertyType);
}
object[] values = new object[properties.Count];
foreach (T item in data)
{
for (int i = 0; i )
{
values[i] = properties[i].GetValue(item);
}
dataTable.Rows.Add(values);
}
return dataTable;
}
///
/// 导出Excel
///
/// 数据源
/// 工作簿Worksheet
/// //是否显示行编号
/// 要导出的列
///
public static byte[] ExportExcel(DataTable dataTable, string heading = "", bool showSrNo = false, params string[] columnsToTake)
{
byte[] result = null;
using(ExcelPackage package=new ExcelPackage())
{
ExcelWorksheet workSheet = package.Workbook.Worksheets.Add(string.Format("{0}Data", heading));
int startRowFrom = string.IsNullOrEmpty(heading) ? 1 : 3; //开始的行
//是否显示行编号
if (showSrNo)
{
DataColumn dataColumn = dataTable.Columns.Add("#", typeof(int));
dataColumn.SetOrdinal(0);
int index = 1;
foreach (DataRow item in dataTable.Rows)
{
item[0] = index;
index++;
}
}
//Add Content Into the Excel File
workSheet.Cells["A" + startRowFrom].LoadFromDataTable(dataTable, true);
// autofit width of cells with small content
int columnIndex = 1;
foreach (DataColumn item in dataTable.Columns)
{
ExcelRange columnCells = workSheet.Cells[workSheet.Dimension.Start.Row, columnIndex, workSheet.Dimension.End.Row, columnIndex];
int maxLength = columnCells.Max(cell => cell.Value.ToString().Count());
if (maxLength 150)
{
workSheet.Column(columnIndex).AutoFit();
}
columnIndex++;
}
// format header - bold, yellow on black
using (ExcelRange r = workSheet.Cells[startRowFrom, 1, startRowFrom, dataTable.Columns.Count])
{
r.Style.Font.Color.SetColor(System.Drawing.Color.White);
r.Style.Font.Bold = true;
r.Style.Fill.PatternType = OfficeOpenXml.Style.ExcelFillStyle.Solid;
r.Style.Fill.BackgroundColor.SetColor(System.Drawing.ColorTranslator.FromHtml("#1fb5ad"));
}
// format cells - add borders
using (ExcelRange r = workSheet.Cells[startRowFrom + 1, 1, startRowFrom + dataTable.Rows.Count, dataTable.Columns.Count])
{
r.Style.Border.Top.Style = ExcelBorderStyle.Thin;
r.Style.Border.Bottom.Style = ExcelBorderStyle.Thin;
r.Style.Border.Left.Style = ExcelBorderStyle.Thin;
r.Style.Border.Right.Style = ExcelBorderStyle.Thin;
r.Style.Border.Top.Color.SetColor(System.Drawing.Color.Black);
r.Style.Border.Bottom.Color.SetColor(System.Drawing.Color.Black);
r.Style.Border.Left.Color.SetColor(System.Drawing.Color.Black);
r.Style.Border.Right.Color.SetColor(System.Drawing.Color.Black);
}
// removed ignored columns
for (int i = dataTable.Columns.Count - 1; i >= 0; i--)
{
if (i == 0 && showSrNo)
{
continue;
}
if (!columnsToTake.Contains(dataTable.Columns[i].ColumnName))
{
workSheet.DeleteColumn(i + 1);
}
}
if (!String.IsNullOrEmpty(heading))
{
workSheet.Cells["A1"].Value = heading;
workSheet.Cells["A1"].Style.Font.Size = 20;
workSheet.InsertColumn(1, 1);
workSheet.InsertRow(1, 1);
workSheet.Column(1).Width = 5;
}
result = package.GetAsByteArray();
}
return result;
}
///
/// 导出Excel
///
///
///
///
///
///
///
public static byte[] ExportExcel(List data, string heading = "", bool isShowSlNo = false, params string[] ColumnsToTake)
{
return ExportExcel(ListToDataTable(data), heading, isShowSlNo, ColumnsToTake);
}
}
3.获取数据并显示
//数据类
public class StaticDataOfStudent
{
public static List ListStudent
{
get
{
return new List()
{
new Student(){ID=1,Name="曹操",Sex="男",Email="caocao@163.com",Age=24},
new Student(){ID=2,Name="李易峰",Sex="女",Email="lilingjie@sina.com.cn",Age=24},
new Student(){ID=3,Name="张三丰",Sex="男",Email="zhangsanfeng@qq.com",Age=224},
new Student(){ID=4,Name="孙权",Sex="男",Email="sunquan@163.com",Age=1224},
};
}
}
}
//用于显示的数据类
public class StudentViewModel
{
public List ListStudent
{
get
{
return StaticDataOfStudent.ListStudent;
}
}
}
实现部分:
1.控制器
public class HomeController : Controller
{
// GET: Home
public ActionResult Index()
{
StudentViewModel model = new StudentViewModel();
return View(model);
}
//导出
public FileContentResult ExportToExcel()
{
List lstStudent = StaticDataOfStudent.ListStudent;
string[] columns = { "ID", "Name","Age"};
byte[] filecontent = ExcelExportHelper.ExportExcel(lstStudent,"", false, columns);
return File(filecontent, ExcelExportHelper.ExcelContentType, "MyStudent.xlsx");
}
}
2.视图
@model ExportToExcel.Models.StudentViewModel
@{
ViewBag.Title = "Excel文件导出";
}
class=
"panel">
class=
"panel-body">
class="table table-striped table-bordered">
ID |
Name |
Sex |
Age |
Email |
@foreach (var item in Model.ListStudent)
{
@item.ID |
@item.Name |
@item.Sex |
@item.Age |
@item.Email |
}
C# 使用Epplus导出数据到Excel
标签:listt 准备 使用 ble 数据源 index summary package tran
原文地址:https://www.cnblogs.com/zhang1f/p/11093679.html