后缀表达式 Java实现
标签:字符串 算法 pos ring expr pre style http sop
基本原理:
从左到右扫描字符串:1、是操作数:压栈。 2、是操作符:出栈两个操作数,将运算结果压栈。
扫描字符串通过java.util.Scanner类实现,其next方法可以读取以空格(默认)或指定符号分割的元素。
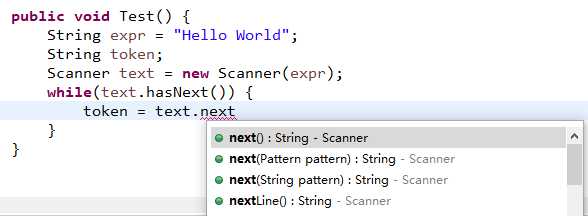
算法代码:
1 public class PostfixEvaluator{
2 private final static char ADD = ‘+‘;
3 private final static char SUBTRACT = ‘-‘;
4 private final static char MULTIPLY = ‘*‘;
5 private final static char DIVIDE = ‘/‘;
6
7 private Stack stack;
8
9 public PostfixEvaluator() {
10 stack = new Stack();
11 }
12
13 public int evaluate(String expr) {
14 int op1,op2,result=0;
15 String token;
16 Scanner parser = new Scanner(expr);
17
18 while(parser.hasNext()) {
19 token = parser.next();
20
21 if(isOperator(token)) {
22 op2 = (stack.pop()).intValue();
23 op1 = (stack.pop()).intValue();
24 result = evaluateSingleOperator(token.charAt(0),op1,op2);
25 stack.push((Integer)result);
26 }else {
27 stack.push(Integer.parseInt(token));
28 }
29 }
30 return result;
31 }
32
33 private boolean isOperator(String token) {
34 return (token.equals("+") || token.equals("-") ||
35 token.equals("*") || token.equals("/"));
36 }
37
38 private int evaluateSingleOperator(char operation,int op1,int op2) {
39 int result = 0;
40 switch(operation) {
41 case ADD:
42 result = op1+op2;
43 break;
44 case SUBTRACT:
45 result = op1-op2;
46 break;
47 case MULTIPLY:
48 result = op1*op2;
49 break;
50 case DIVIDE:
51 result = op1/op2;
52 }
53 return result;
54 }
55 }
后缀表达式 Java实现
标签:字符串 算法 pos ring expr pre style http sop
原文地址:https://www.cnblogs.com/ShadowCharle/p/11167043.html
评论