[Java]数据分析--数据可视化
标签:i++ math pre color nextval 包含 图片 extends public
时间序列
- 需求:将一组字符顺序添加到时间序列中
- 实现:定义时间序列类TimeSeries,包含静态类Entry表示序列类中的各项,以及add,get,iterator,entry方法
TimeSeries.java


1 import java.util.ArrayList;
2 import java.util.Iterator;
3 import java.util.Map;
4 import java.util.TreeMap;
5 import java.util.concurrent.TimeUnit;
6
7 public class TimeSeriesimplements Iterable {
8 private final Map map = new TreeMap();
9
10 public void add(long time, T event) {
11 map.put(time, event);
12 try {
13 TimeUnit.MICROSECONDS.sleep(1); // 0.000001 sec delay
14 } catch(InterruptedException e) {
15 System.err.println(e);
16 }
17 }
18
19 public T get(long time) {
20 return map.get(time);
21 }
22
23 ArrayList getList() {
24 ArrayList list = new ArrayList();
25 for (Entry entry : this) {
26 list.add(entry);
27 }
28 return list;
29 }
30
31 public int size() {
32 return map.size();
33 }
34
35 @Override
36 public Iterator iterator() {
37 return new Iterator() { // anonymous inner class
38 private final Iterator it = map.keySet().iterator();
39
40 @Override
41 public boolean hasNext() {
42 return it.hasNext();
43 }
44
45 @Override
46 public Entry next() {
47 long time = (Long)it.next();
48 T event = map.get(time);
49 return new Entry(time, event);
50 }
51 };
52 }
53
54 public static class Entry {
55 private final Long time;
56 private final T event;
57
58 public Entry(long time, T event) {
59 this.time = time;
60 this.event = event;
61 }
62
63 public long getTime() {
64 return time;
65 }
66
67 public T getEvent() {
68 return event;
69 }
70
71 @Override
72 public String toString() {
73 return String.format("(%d, %s)", time, event);
74 }
75 }
76 }
View Code
TimeSeriesTester.java


1 import java.util.ArrayList;
2
3 public class TimeSeriesTester {
4 final static String[] EVENTS = {"It", "was", "the", "best", "of", "times"};
5
6 public static void main(String[] args) {
7 TimeSeries series = new TimeSeries();
8 load(series);
9
10 for (TimeSeries.Entry entry : series) {
11 long time = entry.getTime();
12 String event = entry.getEvent();
13 System.out.printf("%16d: %s%n", time, event);
14 }
15
16 ArrayList list = series.getList();
17 System.out.printf("list.get(3) = %s%n", list.get(3));
18 }
19
20 static void load(TimeSeries series) {
21 for (String event : EVENTS) {
22 series.add(System.currentTimeMillis(), event);
23 }
24 }
25 }
View Code
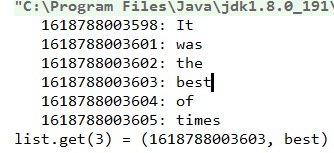
移动平均
- 需求:实现一个序列,其值是原始序列连续片段的平均值
- 实现:定义移动平均类MovingAverage,继承TimeSeries,同时实现根据原始序列和片段长度生成新序列的构造方法,tmp每次计算保存一个片段,sum计算片段元素之和,最后把平均值添加到序列中
- 注意
- 如原始序列有8个元素,片段长度为3,则新序列长度为 8-8%3=6
- 对于a=b=c,先执行b=c,再执行a=b
- tmp不是每次全部更新,替换一个元素即可,求和后i自增
MovingAverage.java


1 import java.util.Iterator;
2
3 public class MovingAverage extends TimeSeries {
4 private final TimeSeries parent;
5 private final int length;
6
7 public MovingAverage(TimeSeries parent, int length) {
8 this.parent = parent;
9 this.length = length;
10 if (length > parent.size()) {
11 throw new IllegalArgumentException("That‘s too long.");
12 }
13
14 double[] tmp = new double[length]; // temp array to compute averages
15 double sum = 0;
16 int i=0;
17 Iterator it = parent.iterator();
18 for (int j = 0; j ) {
19 sum += tmp[i++] = nextValue(it);
20 }
21 this.add(System.currentTimeMillis(), sum/length);
22
23 while (it.hasNext()) {
24 sum -= tmp[i%length];
25 sum += tmp[i++%length] = nextValue(it);
26 this.add(System.currentTimeMillis(), sum/length);
27 }
28 }
29
30 /* Returns the double value in the Entry currently located by it.
31 */
32 private static double nextValue(Iterator it) {
33 TimeSeries.Entry entry = (TimeSeries.Entry)it.next();
34 return entry.getEvent();
35 }
36 }
View Code
MovingAverageTester.java


1 public class MovingAverageTester {
2 static final double[] DATA = {20, 25, 21, 26, 28, 27, 29, 31};
3
4 public static void main(String[] args) {
5 TimeSeries series = new TimeSeries();
6 for (double x : DATA) {
7 series.add(System.currentTimeMillis(), x);
8 }
9 System.out.println(series.getList());
10
11 TimeSeries ma3 = new MovingAverage(series, 3);
12 System.out.println(ma3.getList());
13
14 TimeSeries ma5 = new MovingAverage(series, 5);
15 System.out.println(ma5.getList());
16 }
17 }
View Code

指数分布
- 需求:模拟输出客服接电话的时间,两次电话之间的时间为指数分布,已知平均8小时接到120个电话
- 实现:平均等待时间为4分钟,密度参数为0.25,生成随机数后代入指数分布公式,即得到时间


1 import java.util.Random;
2
3 public class ArrivalTimesTester {
4 static final Random random = new Random();
5 static final double LAMBDA = 0.25;
6
7 static double time() {
8 double p = random.nextDouble();
9 return -Math.log(1 - p)/LAMBDA;
10 }
11
12 public static void main(String[] args) {
13 double sum=0;
14 for (int i = 0; i ) {
15 System.out.println(time());
16 sum += time();
17 }
18 System.out.println("\n");
19 System.out.println(sum/8);
20 }
21 }
View Code
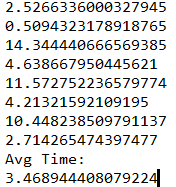
[Java]数据分析--数据可视化
标签:i++ math pre color nextval 包含 图片 extends public
原文地址:https://www.cnblogs.com/cxc1357/p/14675173.html
评论